Astar3D示例代码
时间: 2024-06-16 19:07:22 浏览: 161
Astar3D是一种三维路径规划算法,用于在三维空间中找到最短路径。下面是一个简单的Astar3D示例代码:
```python
class Node:
def __init__(self, x, y, z):
self.x = x
self.y = y
self.z = z
self.g = 0
self.h = 0
self.f = 0
self.parent = None
def astar3d(start, goal):
open_list = []
closed_list = []
open_list.append(start)
while len(open_list) > 0:
current_node = open_list
current_index = 0
for index, node in enumerate(open_list):
if node.f < current_node.f:
current_node = node
current_index = index
open_list.pop(current_index)
closed_list.append(current_node)
if current_node == goal:
path = []
current = current_node
while current is not None:
path.append((current.x, current.y, current.z))
current = current.parent
return path[::-1]
neighbors = get_neighbors(current_node) # 获取当前节点的邻居节点
for neighbor in neighbors:
if neighbor in closed_list:
continue
neighbor.g = current_node.g + distance(current_node, neighbor)
neighbor.h = heuristic(neighbor, goal)
neighbor.f = neighbor.g + neighbor.h
if neighbor in open_list:
if neighbor.g < neighbor.parent.g:
neighbor.parent = current_node
else:
neighbor.parent = current_node
open_list.append(neighbor)
return None
def distance(node1, node2):
# 计算两个节点之间的距离
# 这里可以根据实际情况使用不同的距离计算方法,如欧几里得距离、曼哈顿距离等
return math.sqrt((node1.x - node2.x)**2 + (node1.y - node2.y)**2 + (node1.z - node2.z)**2)
def heuristic(node, goal):
# 估算当前节点到目标节点的距离
# 这里可以根据实际情况使用不同的估算方法,如欧几里得距离、曼哈顿距离等
return math.sqrt((node.x - goal.x)**2 + (node.y - goal.y)**2 + (node.z - goal.z)**2)
def get_neighbors(node):
# 获取当前节点的邻居节点
# 这里可以根据实际情况定义邻居节点的获取方式,如八个方向、六个方向等
neighbors = []
# ...
return neighbors
# 示例用法
start_node = Node(0, 0, 0)
goal_node = Node(5, 5, 5)
path = astar3d(start_node, goal_node)
print(path)
```
这段示例代码实现了一个简单的Astar3D算法,其中包括了节点类Node的定义,Astar3D算法的主要逻辑函数astar3d,以及一些辅助函数。你可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
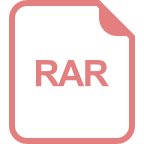

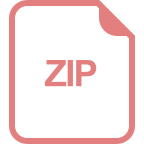
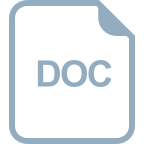
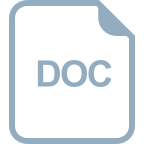
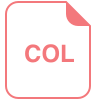
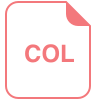


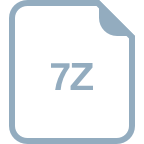
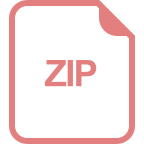
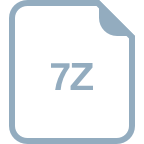