A*算法解决迷宫问题
时间: 2023-11-28 09:44:06 浏览: 98
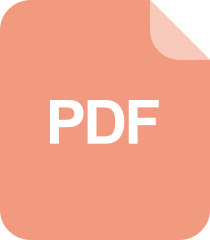
A_star求解迷宫问题.pdf
A*算法是一种启发式搜索算法,可以用于解决迷宫问题。它通过估计从起点到终点的距离来指导搜索方向,从而提高搜索效率。下面是使用A*算法解决迷宫问题的步骤:
1. 定义状态表示:将迷宫看作一个二维网格图,每个格子表示一个状态,状态包括格子的坐标、是否可达、起点到该格子的距离和该格子到终点的估计距离(即启发函数值)。
2. 定义启发函数:启发函数用于估计从当前状态到达终点的距离,常用的启发函数有曼哈顿距离和欧几里得距离。
3. 定义开放列表和关闭列表:开放列表用于存储待扩展的状态,关闭列表用于存储已扩展的状态。
4. 初始化:将起点加入开放列表,并将起点到起点的距离设为0,起点到终点的估计距离设为启发函数值。
5. 迭代扩展状态:重复以下步骤直到找到终点或开放列表为空:
1)从开放列表中选取启发函数值最小的状态作为当前状态。
2)将当前状态从开放列表中移除,并加入关闭列表。
3)如果当前状态为终点,则搜索结束。
4)否则,将当前状态的相邻可达状态加入开放列表,并更新它们的起点到起点的距离和该状态到终点的估计距离。
6. 回溯路径:从终点开始,沿着每个状态的父状态指针回溯到起点,即可得到一条最短路径。
下面是一个使用A*算法解决迷宫问题的Python代码示例:
```python
import heapq
def manhattan_distance(point1, point2):
return abs(point1[0] - point2[0]) + abs(point1[1] - point2[1])
def astar(maze, start, end):
rows, cols = len(maze), len(maze[0])
open_list = [(0, start)]
closed_list = set()
parent = {}
g_score = {start: 0}
f_score = {start: manhattan_distance(start, end)}
while open_list:
_, current = heapq.heappop(open_list)
if current == end:
path = []
while current in parent:
path.append(current)
current = parent[current]
path.append(start)
return path[::-1]
closed_list.add(current)
for i, j in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
neighbor = current[0] + i, current[1] + j
if 0 <= neighbor[0] < rows and 0 <= neighbor[1] < cols and maze[neighbor[0]][neighbor[1]] == 0:
tentative_g_score = g_score[current] + 1
if neighbor in closed_list and tentative_g_score >= g_score.get(neighbor, float('inf')):
continue
if tentative_g_score < g_score.get(neighbor, float('inf')):
parent[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = tentative_g_score + manhattan_distance(neighbor, end)
heapq.heappush(open_list, (f_score[neighbor], neighbor))
return None
maze = [[0, 0, 0, 0, 0],
[0, 1, 1, 0, 0],
[0, 0, 0, 0, 0],
[0, 1, 1, 1, 0],
[0, 0, 0, 0, 0]]
start = (0, 0)
end = (4, 4)
path = astar(maze, start, end)
print(path)
```
阅读全文
相关推荐
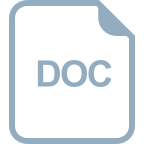
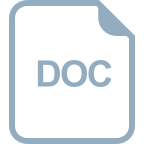
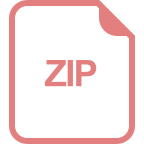

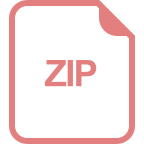
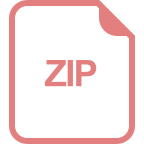
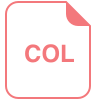


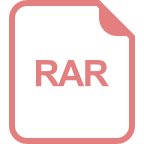
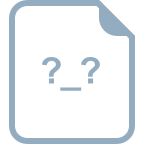
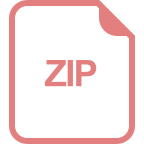
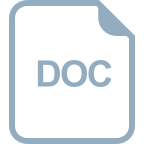


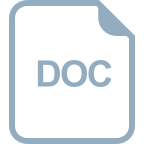