用c++实现矩阵乘法,并分析时间复杂度
时间: 2024-10-26 09:02:29 浏览: 46
在C++中实现矩阵乘法,最常见的是采用两层嵌套循环的方法,也称为“标准算法”或“列主序算法”。下面是简单的矩阵乘法示例:
```cpp
#include <iostream>
// 矩阵类模板
template <typename T>
class Matrix {
public:
int rows;
int cols;
T data[/*rows * cols*/];
// 构造函数
Matrix(int r, int c) : rows(r), cols(c) {}
// 矩阵乘法运算
void multiply(const Matrix<T>& other) {
if (cols != other.rows) {
std::cerr << "Matrix dimensions don't match for multiplication." << std::endl;
return;
}
T result[rows][other.cols];
for (int i = 0; i < rows; ++i)
for (int j = 0; j < other.cols; ++j)
for (int k = 0; k < cols; ++k)
result[i][j] += data[i * cols + k] * other.data[k * other.cols + j];
// 将结果赋给当前矩阵
for (int i = 0; i < rows; ++i)
for (int j = 0; j < other.cols; ++j)
data[i * cols + j] = result[i][j];
}
// 显示矩阵内容
void display() const {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j)
std::cout << data[i * cols + j] << ' ';
std::cout << '\n';
}
}
};
int main() {
Matrix<int> m(2, 3);
Matrix<int> n(3, 4);
// 初始化矩阵...
m.display(); // 输出m
n.display(); // 输出n
m.multiply(n); // 计算并存储mn的结果
m.display(); // 输出结果矩阵mn
return 0;
}
```
**时间复杂度分析**:
矩阵乘法的时间复杂度取决于输入矩阵的维度。标准算法的时间复杂度是O(n^3),其中n是矩阵的行数或列数。这是因为我们需要对每个元素进行三次迭代(两个外层循环遍历行和列,内层循环计算对应位置的乘积)。这是由于乘法操作的数量是O(n^2),再加上需要遍历所有元素,所以总的时间复杂度是O(n^3)。在实际应用中,如果矩阵非常大,这种算法可能会显得效率较低。
阅读全文
相关推荐
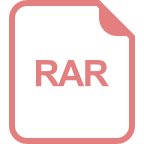
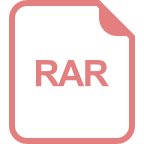
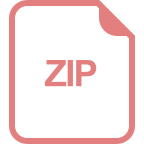
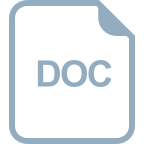

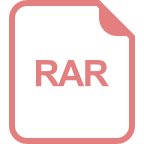
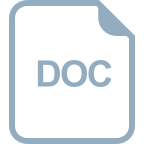
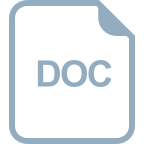

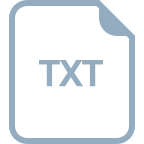
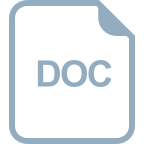
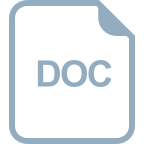
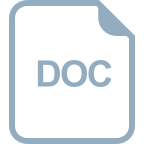
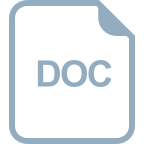
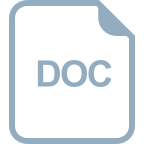
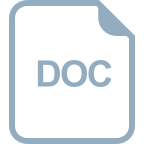


