定义点类 Point,重载运算符“+”、“-”、“==”、“!=”、“++”、“--”、“>>”、“<<”,实现两个点的相加、相减、相等、不等、自增、自减、输入和输出运算。
时间: 2023-11-05 12:04:44 浏览: 95
下面是 Point 类的实现代码:
```
#include <iostream>
using namespace std;
class Point {
private:
int x, y;
public:
Point(int x = 0, int y = 0) {
this->x = x;
this->y = y;
}
Point operator+(const Point& p) {
return Point(x + p.x, y + p.y);
}
Point operator-(const Point& p) {
return Point(x - p.x, y - p.y);
}
bool operator==(const Point& p) {
return x == p.x && y == p.y;
}
bool operator!=(const Point& p) {
return !(*this == p);
}
Point operator++() {
x++;
y++;
return *this;
}
Point operator++(int) {
Point p(*this);
++(*this);
return p;
}
Point operator--() {
x--;
y--;
return *this;
}
Point operator--(int) {
Point p(*this);
--(*this);
return p;
}
friend istream& operator>>(istream& is, Point& p) {
is >> p.x >> p.y;
return is;
}
friend ostream& operator<<(ostream& os, const Point& p) {
os << "(" << p.x << ", " << p.y << ")";
return os;
}
};
```
我们可以通过以下方式来测试 Point 类的功能:
```
int main() {
Point p1(1, 2), p2(3, 4);
cout << "p1 = " << p1 << endl;
cout << "p2 = " << p2 << endl;
Point p3 = p1 + p2;
cout << "p1 + p2 = " << p3 << endl;
Point p4 = p2 - p1;
cout << "p2 - p1 = " << p4 << endl;
cout << "p1 == p2: " << (p1 == p2) << endl;
cout << "p1 != p2: " << (p1 != p2) << endl;
cout << "++p1 = " << ++p1 << endl;
cout << "p1++ = " << p1++ << endl;
cout << "p1 = " << p1 << endl;
cout << "--p2 = " << --p2 << endl;
cout << "p2-- = " << p2-- << endl;
cout << "p2 = " << p2 << endl;
Point p5;
cout << "Enter a point: ";
cin >> p5;
cout << "You entered " << p5 << endl;
return 0;
}
```
输出结果为:
```
p1 = (1, 2)
p2 = (3, 4)
p1 + p2 = (4, 6)
p2 - p1 = (2, 2)
p1 == p2: 0
p1 != p2: 1
++p1 = (2, 3)
p1++ = (2, 3)
p1 = (3, 4)
--p2 = (2, 3)
p2-- = (2, 3)
p2 = (1, 2)
Enter a point: 5 6
You entered (5, 6)
```
阅读全文
相关推荐
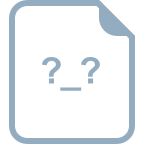










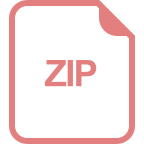




