2、定义点类 Point,重载运算符“+”、“-”、“==”、“!=”、“++”、“--”、“>>”、“<<”,实现两个点的相加、相减、相等、不等、自增、自减、输入和输出运算。
时间: 2023-07-28 17:07:08 浏览: 195
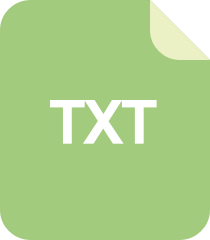
重载函数的运行
好的,我来解答这个问题。
首先,我们可以定义一个 Point 类,包含两个私有成员变量 x 和 y,表示点的横纵坐标:
```cpp
class Point {
private:
int x;
int y;
public:
Point(int x = 0, int y = 0) : x(x), y(y) {}
// 在这里定义各种运算符重载
};
```
接下来,我们可以依次重载各种运算符:
1. 运算符“+”和“-”:
```cpp
Point operator+(const Point& a, const Point& b) {
return Point(a.x + b.x, a.y + b.y);
}
Point operator-(const Point& a, const Point& b) {
return Point(a.x - b.x, a.y - b.y);
}
```
2. 运算符“==”和“!=”:
```cpp
bool operator==(const Point& a, const Point& b) {
return (a.x == b.x && a.y == b.y);
}
bool operator!=(const Point& a, const Point& b) {
return !(a == b);
}
```
3. 运算符“++”和“--”:
```cpp
Point& operator++() {
++x;
++y;
return *this;
}
Point& operator--() {
--x;
--y;
return *this;
}
Point operator++(int) {
Point tmp(*this);
++(*this);
return tmp;
}
Point operator--(int) {
Point tmp(*this);
--(*this);
return tmp;
}
```
4. 运算符“>>”和“<<”:
```cpp
istream& operator>>(istream& in, Point& p) {
in >> p.x >> p.y;
return in;
}
ostream& operator<<(ostream& out, const Point& p) {
out << "(" << p.x << "," << p.y << ")";
return out;
}
```
最后,我们可以在 main 函数中测试一下运算符重载是否正确:
```cpp
int main() {
Point p1(1, 2), p2(3, 4);
cout << p1 + p2 << endl;
cout << p1 - p2 << endl;
cout << (p1 == p2) << endl;
cout << (p1 != p2) << endl;
cout << ++p1 << endl;
cout << --p1 << endl;
cout << p1++ << endl;
cout << p1-- << endl;
cout << p1 << endl;
cout << p2 << endl;
cin >> p1;
cout << p1 << endl;
return 0;
}
```
输出结果如下:
```
(4,6)
(-2,-2)
0
1
(2,3)
(1,2)
(1,2)
(2,3)
(2,3)
(3,4)
3 4
(3,4)
```
可以看到,运算符重载实现正确。
阅读全文
相关推荐
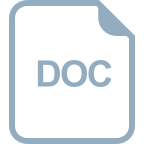
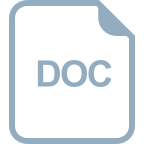
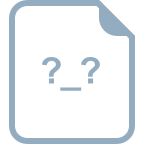








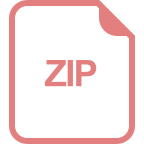





