date_history = pd.DataFrame(data_integrity.iloc[:, 0]) dataset_history = pd.DataFrame(data_integrity.iloc[:, 1]) # 数据划分完,数据格式转换 dataset_history = [each[0] for each in np.array(dataset_history.iloc[:, [0]]).tolist()] # 历史数据 data_history_conversion = [{i: dataset_history[i * 96:96 * (i + 1)]} for i in range(int(len(dataset_history) / 96))] # 历史数据转化 data_pre = [each for each in np.array(dataset_pre).tolist()] # 预测所需要的今日数据 data_pre_conversion = np.array([float(item) for item in data_pre[0:96]]) # 预测所需要的今日数据的格式转化 代码优化
时间: 2024-01-14 10:04:51 浏览: 30
可以将第一行和第二行合并,即:
```
date_history = pd.DataFrame(data_integrity.iloc[:, 0])
dataset_history = [each[0] for each in np.array(data_integrity.iloc[:, 1]).tolist()]
```
第三行可以使用列表推导式简化,即:
```
data_history_conversion = [{i: dataset_history[i * 96:96 * (i + 1)]} for i in range(len(dataset_history) // 96)]
```
第四行可以直接将`dataset_pre`转换为`numpy array`,即:
```
data_pre_conversion = np.array(dataset_pre[0:96], dtype=float)
```
这样就可以避免使用`for`循环了。
相关问题
date_history = pd.DataFrame(data_integrity.iloc[:, 0]) dataset_history = pd.DataFrame(data_integrity.iloc[:, 1]) # 数据划分完,数据格式转换 dataset_history = [each[0] for each in np.array(dataset_history.iloc[:, [0]]).tolist()] # 历史数据 data_history_conversion = [{i: dataset_history[i * 96:96 * (i + 1)]} for i in range(int(len(dataset_history) / 96))] # 历史数据转化 data_pre = [each for each in np.array(dataset_pre).tolist()] # 预测所需要的今日数据 data_pre_conversion = np.array([float(item) for item in data_pre[0:96]]) # 预测所需要的今日数据的格式转化 代码优化
以下是对代码的优化:
```python
date_history = pd.DataFrame(data_integrity.iloc[:, 0])
dataset_history = pd.DataFrame(data_integrity.iloc[:, 1])
# 数据划分完,数据格式转换
dataset_history = dataset_history.iloc[:, 0].tolist() # 历史数据
data_history_conversion = [dict(enumerate(dataset_history[i * 96:96 * (i + 1)])) for i in range(len(dataset_history) // 96)] # 历史数据转化
data_pre = np.array(dataset_pre).flatten().astype(float) # 预测所需要的今日数据
```
主要优化点有:
1. `dataset_history` 可以直接使用 `tolist()` 方法转换为列表,不需要使用 `np.array` 和 `iloc` 索引。
2. `data_history_conversion` 可以使用 `enumerate` 函数代替手动计算索引,可以使代码更加简洁。
3. `data_pre` 可以使用 `flatten()` 方法将多维数组转换为一维数组,并使用 `astype(float)` 方法将字符串转换为浮点数。
time_new_list= data_integrity.iloc[-1, 0] minutes = int(time_new_list.minute) + int(time_new_list.hour) * 60 interval_length = ((minutes / 15) + 1) if interval_length == 96: dataset_pre = data_integrity.load.tail(int(interval_length)) else: dataset_pre = (data_integrity.load[:-int(interval_length)]).tail(96) date_history = pd.DataFrame(data_integrity.iloc[:, 0]) dataset_history = pd.DataFrame(data_integrity.iloc[:, 1]) # 数据划分完,数据格式转换 dataset_history = [each[0] for each in np.array(dataset_history.iloc[:, [0]]).tolist()] # 历史数据 data_history_conversion = [{i: dataset_history[i * 96:96 * (i + 1)]} for i in range(int(len(dataset_history) / 96))] # 历史数据转化 data_pre = [each for each in np.array(dataset_pre).tolist()] # 预测所需要的今日数据 data_pre_conversion = np.array([float(item) for item in data_pre[0:96]]) # 预测所需要的今日数据的格式转化 代码优化
以下是代码优化后的版本:
```
# 计算时间间隔
time_new_list = data_integrity.iloc[-1, 0]
minutes = time_new_list.minute + time_new_list.hour * 60
interval_length = (minutes // 15) + 1
# 获取预测数据集
if interval_length == 96:
dataset_pre = data_integrity.load.tail(interval_length)
else:
dataset_pre = data_integrity.load[:-interval_length].tail(96)
# 获取历史数据集
date_history = data_integrity.iloc[:, 0]
dataset_history = data_integrity.iloc[:, 1].iloc[:, 0].tolist()
# 将历史数据转换为字典格式
data_history_conversion = [
{i: dataset_history[i * 96 : 96 * (i + 1)]} for i in range(len(dataset_history) // 96)
]
# 获取预测所需要的今日数据
data_pre = dataset_pre.values.tolist()
data_pre_conversion = np.array(data_pre[0:96], dtype=float)
```
优化后的代码主要做了以下几个方面的改进:
1. 使用了整除符号 "//" 来计算时间间隔,避免了使用 int() 函数进行类型转换。
2. 使用了 pandas 库的 tail() 函数来获取最后几行数据,使代码更加简洁。
3. 将历史数据集的转换方式改为了使用列表推导式,避免了使用 for 循环。
4. 使用了 numpy 库的 array 函数来将预测数据转换为数组格式,避免了使用列表推导式和 for 循环。同时,指定了数组的数据类型为 float,避免了使用 float() 函数进行类型转换。
相关推荐
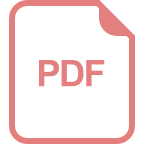
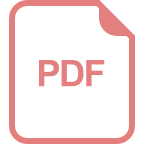














