编写一个C 程序,并使用系统调用fork()创建一个子进程。要求如下: ①在子进程中分别输出当前进程为子进程的提示、当前进程的PID 和父进程的PID、根据用户输入确定当前进程的返回值、退出提示等信息。 ②在父进程中分别输出当前进程为父进程的提示、当前进程的PID 和子进程的PID、等待子进程退出后获得的返回值、退出提示等信息。
时间: 2024-02-06 21:11:31 浏览: 101
好的,我理解了你的要求,以下是实现该功能的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
int status;
pid = fork();
if (pid < 0) { // fork失败
printf("Fork failed\n");
exit(1);
} else if (pid == 0) { // 子进程
printf("This is child process, PID: %d, Parent PID: %d\n", getpid(), getppid());
int ret;
printf("Please enter a return value for child process: ");
scanf("%d", &ret);
printf("Child process will return %d\n", ret);
exit(ret);
} else { // 父进程
printf("This is parent process, PID: %d, Child PID: %d\n", getpid(), pid);
wait(&status);
if (WIFEXITED(status)) { // 子进程正常退出
printf("Child process returned %d\n", WEXITSTATUS(status));
} else { // 子进程异常退出
printf("Child process terminated abnormally\n");
}
printf("Parent process is exiting\n");
}
return 0;
}
```
这个程序中,我们首先使用fork()创建了一个子进程,然后在子进程中输出了当前进程的提示、PID和父进程的PID,接着通过用户输入确定了当前进程的返回值,并通过exit()函数退出。在父进程中,我们输出了当前进程的提示、PID和子进程的PID,然后使用wait()函数等待子进程退出,并通过WIFEXITED和WEXITSTATUS宏判断子进程是正常退出还是异常退出,并输出相应的信息。最后,父进程退出。
阅读全文
相关推荐
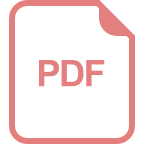
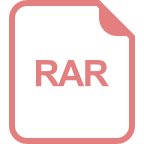
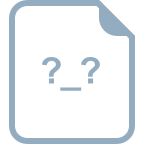















