采用Verilog设计简单的红绿灯。 绿灯20s;黄灯5s;红灯20s。 已知系统时钟clk=1MHz 要求: (1)设计分频模块;计数器模块。 (2)顶层模块引用分频模块和计数模块。
时间: 2024-10-19 21:14:10 浏览: 21
在Verilog设计中创建一个简单的红绿灯控制器,我们可以采用状态机模型来实现。首先,我们需要几个主要部分:
1. **分频模块**(Divider Module):
设计一个分频器用于将1MHz的时钟频率降低到我们需要的周期时间(例如25秒总周期= 20s绿灯+5s黄灯+20s红灯)。我们可以使用寄存器和减法器来实现这个功能,通过将时钟周期数除以需要的周期数。
```verilog
module divide_module(
input clk,
input [7:0] desired_period, // 16位表示2^16个时钟周期
output reg [15:0] current_counter
);
...
always @(posedge clk) begin
if (current_counter == desired_period - 1) begin
current_counter <= 0; // 当计数满后重置
} else {
current_counter <= current_counter + 1;
}
end
...
endmodule
```
2. **计数器模块**(Counter Module):
使用计数器模块来控制绿、黄、红灯的状态,通常使用三个独立的计数器分别对应绿灯、黄灯和红灯的时间。
```verilog
module traffic_light_counter (
input clk,
input wire green_start, yellow_start, red_start,
output reg green_light, yellow_light, red_light
);
...
always @(posedge clk) begin
if (green_start) begin
green_light <= 1'b1; // 绿灯亮起
yellow_light <= 1'b0;
red_light <= 1'b0;
green_counter <= #20 0; // 开始绿灯阶段,延时20个时钟周期
end else if (yellow_start) begin
green_light <= 1'b0;
yellow_light <= 1'b1; // 黄灯亮起
red_light <= 1'b0;
yellow_counter <= #5 0; // 延迟5个时钟周期
end else if (red_start) begin
green_light <= 1'b0;
yellow_light <= 1'b0;
red_light <= 1'b1; // 红灯亮起
red_counter <= #20 0; // 延迟20个时钟周期
end
end
...
endmodule
```
3. **顶层模块**(Top Module):
引入分频模块和两个计数器模块,并根据绿黄红灯的切换逻辑启动它们。
```verilog
module top_module (
input clk,
input green_start, yellow_start, red_start,
output green_light, yellow_light, red_light
);
...
divide_module divider(clk, 4800, counter_period); // 分频25秒,假设每个颜色20s + 5s
traffic_light_counter green_counter(clk, divider.current_counter, green_light, yellow_light, red_light);
traffic_light_counter yellow_counter(clk, divider.current_counter, ..., yellow_light, ...); // 假设 Yellow Counter 也按类似逻辑设置
traffic_light_counter red_counter(clk, divider.current_counter, ..., red_light, ...);
...
endmodule
```
阅读全文
相关推荐
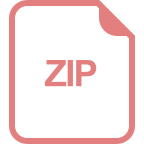
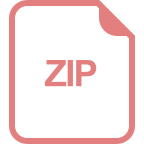
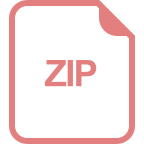


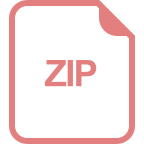
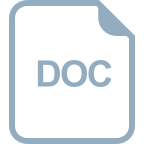












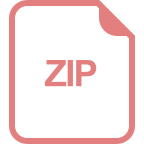