AttributeError: 'NoneType' object has no attribute 'SaveAs'
时间: 2023-10-24 14:06:16 浏览: 36
This error occurs when you try to call the SaveAs method on an object that is None. In other words, the object does not exist or has not been initialized correctly.
To fix this error, you need to make sure that the object you are calling SaveAs on is not None. Check if the object has been initialized correctly and ensure that it is not empty before calling any methods on it.
You can also add a condition to check if the object is None before calling the SaveAs method. For example:
if obj is not None:
obj.SaveAs(filename)
This way, the SaveAs method will only be called if the object is not None.
相关问题
'NoneType' object has no attribute 'SaveAs'
问题中提到的错误"'NoneType' object has no attribute 'SaveAs'"通常是由于对象为None而不是有效对象导致的。这个错误可能是由于以下原因之一引起的:
1. 对象未正确初始化或赋值为None。
2. 对象的属性或方法名称拼写错误。
3. 对象的属性或方法在特定情况下可能为None。
根据提供的引用内容,这个错误可能是由于代码中的driver对象未成功打开浏览器导致的。可能的原因包括:
1. 没有正确添加浏览器驱动程序。
2. 添加的浏览器驱动程序与浏览器版本不兼容。
为了解决这个问题,你可以尝试以下步骤:
1. 确保你已经正确添加了浏览器驱动程序。你可以从官方网站下载适用于你的浏览器版本的驱动程序,并将其放置在正确的位置。
2. 确保驱动程序的版本与你的浏览器版本兼容。如果不兼容,尝试下载适用于你的浏览器版本的驱动程序。
3. 确保你的代码中正确地初始化和使用了driver对象。检查代码中是否存在拼写错误或其他语法错误。
4. 如果问题仍然存在,尝试使用不同的浏览器驱动程序或浏览器版本进行测试,看看是否能够解决问题。
希望这些建议能够帮助你解决问题。如果问题仍然存在,请提供更多的上下文或代码示例,以便我们能够更好地帮助你解决问题。
#### 引用[.reference_title]
- *1* [图片格式导致的报错:Attribute Error: ‘NoneType‘ object has no attribute ‘astype‘ 解决方案](https://blog.csdn.net/qq236237606/article/details/127783866)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [为什么会出现这个?'NoneType' object has no attribute 'contet](https://blog.csdn.net/weixin_33925615/article/details/114409982)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [pycharm中使用pytest运行报错AttributeError: ‘NoneType‘ object has no attribute ‘save_screenshot](https://blog.csdn.net/BaiXuePrincess/article/details/114284058)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
win32com模块来操作Excel文件报错AttributeError: 'NoneType' object has no attribute 'Save'
这个错误通常表示您没有正确地打开Excel文件。请确保您已经成功地使用win32com打开了Excel文件,并且将其分配给了一个变量。如果文件未正确打开,则尝试使用完整路径或检查文件是否存在。
以下是一个简单的例子,演示如何使用win32com打开和保存Excel文件:
```python
import win32com.client as win32
# 打开Excel应用程序
excel = win32.gencache.EnsureDispatch('Excel.Application')
# 打开Excel文件
wb = excel.Workbooks.Open(r'C:\path\to\your\file.xlsx')
# 获取第一个工作表
ws = wb.Worksheets(1)
# 在A1单元格中写入值
ws.Range("A1").Value = "Hello World"
# 保存并关闭Excel文件
wb.Save()
excel.Quit()
```
请注意,如果您在打开文件时遇到错误,可以使用try-except块来捕获错误并忽略保存操作:
```python
try:
wb.Save()
except AttributeError:
pass
excel.Quit()
```
相关推荐
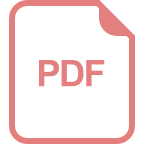
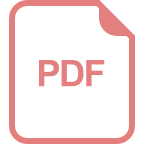
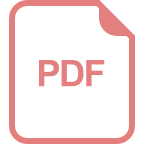









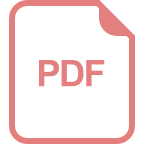
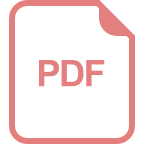
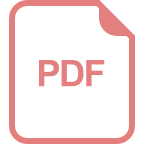
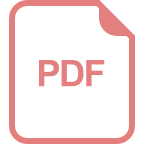