stl queue循环
时间: 2023-09-27 15:03:58 浏览: 118
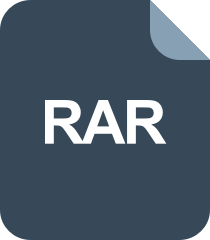
STL.rar_stl queue
STL中的queue不支持循环队列,它是基于单向链表或双向链表实现的,因此只能在队尾插入元素,在队首删除元素。如果需要实现循环队列,可以使用STL中的deque(双端队列),或者自己实现一个循环队列。以下是一个简单的循环队列的实现示例:
```c++
#include <iostream>
#include <vector>
using namespace std;
class CircularQueue {
private:
vector<int> data;
int head;
int tail;
int size;
public:
CircularQueue(int k) {
data.resize(k);
head = -1;
tail = -1;
size = k;
}
bool enQueue(int value) {
if (isFull()) {
return false;
}
if (isEmpty()) {
head = 0;
}
tail = (tail + 1) % size;
data[tail] = value;
return true;
}
bool deQueue() {
if (isEmpty()) {
return false;
}
if (head == tail) {
head = -1;
tail = -1;
return true;
}
head = (head + 1) % size;
return true;
}
int Front() {
if (isEmpty()) {
return -1;
}
return data[head];
}
int Rear() {
if (isEmpty()) {
return -1;
}
return data[tail];
}
bool isEmpty() {
return head == -1;
}
bool isFull() {
return ((tail + 1) % size) == head;
}
};
int main() {
CircularQueue q(5);
cout << q.enQueue(1) << endl; // true
cout << q.enQueue(2) << endl; // true
cout << q.enQueue(3) << endl; // true
cout << q.enQueue(4) << endl; // true
cout << q.enQueue(5) << endl; // true
cout << q.enQueue(6) << endl; // false
cout << q.Front() << endl; // 1
cout << q.Rear() << endl; // 5
cout << q.isFull() << endl; // true
cout << q.deQueue() << endl; // true
cout << q.deQueue() << endl; // true
cout << q.enQueue(6) << endl; // true
cout << q.Front() << endl; // 3
cout << q.Rear() << endl; // 6
return 0;
}
```
这个循环队列使用了一个vector来存储队列元素,head和tail分别表示队头和队尾的下标,size表示队列的大小。当队列满时,tail会指向队列最后一个元素,此时再插入元素就会导致队列溢出。为了避免这种情况,我们使用了取模运算来实现循环队列。
阅读全文
相关推荐
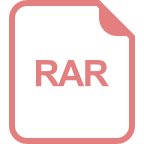
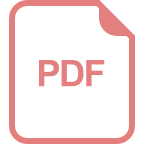

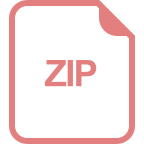
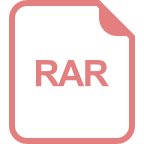
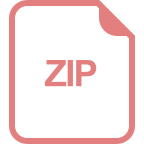
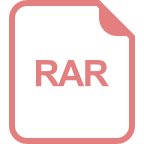
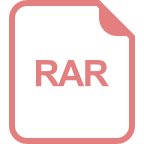
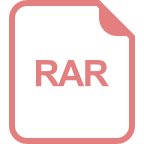
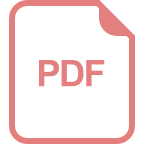
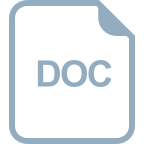
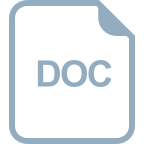
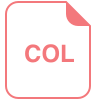




