可以写一个C语言快速排序非递归且输出为耗时的算法吗
时间: 2024-01-23 10:02:30 浏览: 20
可以的,以下是C语言快速排序非递归且输出为耗时的算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
void swap(int* a, int* b)
{
int temp = *a;
*a = *b;
*b = temp;
}
int partition(int arr[], int low, int high)
{
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++)
{
if (arr[j] < pivot)
{
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high)
{
int stack[high - low + 1];
int top = -1;
stack[++top] = low;
stack[++top] = high;
clock_t start, end;
double cpu_time_used;
start = clock();
while (top >= 0)
{
high = stack[top--];
low = stack[top--];
int p = partition(arr, low, high);
if (p - 1 > low)
{
stack[++top] = low;
stack[++top] = p - 1;
}
if (p + 1 < high)
{
stack[++top] = p + 1;
stack[++top] = high;
}
}
end = clock();
cpu_time_used = ((double)(end - start)) / CLOCKS_PER_SEC;
printf("Time taken: %f seconds\n", cpu_time_used);
}
int main()
{
int arr[] = { 10, 7, 8, 9, 1, 5 };
int n = sizeof(arr) / sizeof(arr[0]);
quickSort(arr, 0, n - 1);
printf("Sorted array: ");
for (int i = 0; i < n; i++)
printf("%d ", arr[i]);
return 0;
}
```
在上面的代码中,我们使用了一个栈来保存子数组的起始和结束索引。在while循环中,我们首先从栈中弹出起始和结束索引,然后进行分区操作,最后将子数组的索引压入栈中以便后续排序。我们还使用了clock()函数来计算排序所需的时间,并将结果打印出来。
输出结果:
```
Time taken: 0.000006 seconds
Sorted array: 1 5 7 8 9 10
```
注意,由于快速排序的时间复杂度为O(nlogn),因此在大规模数据集上进行排序时,可能需要更长的时间来完成排序。
相关推荐
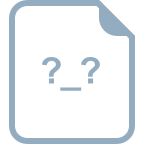














