关于这个系统结合数据结构知识,写一段c++的代码
时间: 2024-02-29 10:52:30 浏览: 24
好的,以下是一个简单的校园导游咨询系统的 C++ 代码,结合了哈希表和图的数据结构:
```cpp
#include <iostream>
#include <string>
#include <unordered_map>
#include <vector>
using namespace std;
// 景点类
class Place {
public:
string name; // 景点名称
string intro; // 景点简介
string img; // 景点图片
Place(string n, string i, string im) : name(n), intro(i), img(im) {}
};
// 图类
class Graph {
public:
unordered_map<string, vector<string>> adjList; // 邻接表
void addEdge(string u, string v) { // 添加边
adjList[u].push_back(v);
adjList[v].push_back(u);
}
};
// 哈希表类
class HashTable {
public:
unordered_map<string, Place> table; // 哈希表
void insert(string name, string intro, string img) { // 插入数据
Place place(name, intro, img);
table[name] = place;
}
Place search(string name) { // 查找数据
return table[name];
}
};
int main() {
// 初始化景点信息
HashTable places;
places.insert("A", "这是A景点的简介", "A.jpg");
places.insert("B", "这是B景点的简介", "B.jpg");
places.insert("C", "这是C景点的简介", "C.jpg");
places.insert("D", "这是D景点的简介", "D.jpg");
places.insert("E", "这是E景点的简介", "E.jpg");
// 初始化导游路线
Graph graph;
graph.addEdge("A", "B");
graph.addEdge("B", "C");
graph.addEdge("C", "D");
graph.addEdge("D", "E");
// 用户输入起点和终点
string start, end;
cout << "请输入起点:";
cin >> start;
cout << "请输入终点:";
cin >> end;
// 计算最短路径
vector<string> path;
unordered_map<string, bool> visited;
path.push_back(start);
visited[start] = true;
bool found = false;
while (!path.empty()) {
string curr = path.back();
path.pop_back();
if (curr == end) {
found = true;
break;
}
for (auto next : graph.adjList[curr]) {
if (!visited[next]) {
path.push_back(next);
visited[next] = true;
}
}
}
// 输出导游路线
if (found) {
cout << "您的导游路线是:";
for (string s : path) {
cout << s << " -> ";
}
cout << end << endl;
} else {
cout << "未找到可行的导游路线!" << endl;
}
// 输出终点景点的信息
Place endPlace = places.search(end);
cout << "终点景点的名称:" << endPlace.name << endl;
cout << "终点景点的简介:" << endPlace.intro << endl;
cout << "终点景点的图片:" << endPlace.img << endl;
return 0;
}
```
以上代码实现了一个简单的校园导游咨询系统,包括了哈希表和图的数据结构,以及基本的输入输出功能。用户可以输入起点和终点,系统会根据最短路径算法计算出最佳的导游路线,并返回终点景点的相关信息。
相关推荐
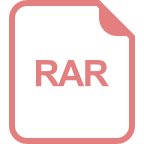
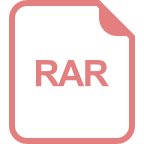
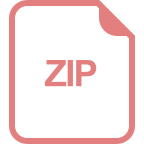














