邻接表求顶点的度C语言
时间: 2023-12-20 19:30:53 浏览: 90
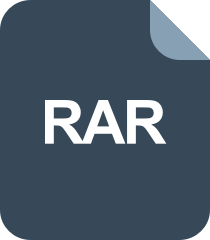
基于邻接边表实现图的顶点结构算法(java源码)
以下是使用邻接表求顶点度数的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTEX_NUM 100 // 最大顶点数
// 边表结点
typedef struct ArcNode {
int adjvex; // 邻接点在顶点数组中的下标
struct ArcNode *nextarc; // 指向下一个邻接点的指针
} ArcNode;
// 顶点表结点
typedef struct VNode {
int data; // 顶点数据
ArcNode *firstarc; // 指向第一个邻接点的指针
} VNode, AdjList[MAX_VERTEX_NUM];
// 图
typedef struct {
AdjList vertices; // 顶点数组
int vexnum, arcnum; // 顶点数和边数
} ALGraph;
// 初始化邻接表
void InitGraph(ALGraph *G, int n) {
G->vexnum = n;
G->arcnum = 0;
for (int i = 0; i < n; i++) {
G->vertices[i].data = i; // 顶点数据为下标
G->vertices[i].firstarc = NULL; // 初始化邻接表为空
}
}
// 添加边
void AddArc(ALGraph *G, int i, int j) {
ArcNode *p = (ArcNode *)malloc(sizeof(ArcNode));
p->adjvex = j;
p->nextarc = G->vertices[i].firstarc;
G->vertices[i].firstarc = p;
G->arcnum++;
}
// 求顶点的度数
int GetDegree(ALGraph *G, int v) {
int degree = 0;
for (ArcNode *p = G->vertices[v].firstarc; p != NULL; p = p->nextarc) {
degree++;
}
return degree;
}
// 主函数
int main() {
int n = 5; // 顶点数
int m = 6; // 边数
int edges[][2] = {{0, 1}, {0, 2}, {1, 2}, {1, 3}, {2, 3}, {3, 4}}; // 边的起点和终点
ALGraph G;
InitGraph(&G, n);
for (int i = 0; i < m; i++) {
AddArc(&G, edges[i][0], edges[i][1]);
AddArc(&G, edges[i][1], edges[i][0]); // 无向图需要添加反向边
}
int v = 2; // 求顶点2的度数
int degree = GetDegree(&G, v);
printf("Vertex %d's degree is %d\n", v, degree);
return 0;
}
```
阅读全文
相关推荐
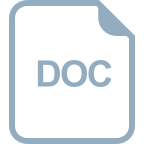














