用遗传算法求解巡航高度优化问题,一个航段只能选择一个高度,将适应度值设置为各个航段的油耗之和,输出每次迭代对应的结果,并绘制适应度值、适应度平均值随迭代次数的变化图。共有三个高度选项,分别为9200、9800、10400米;共有八个航段;第一个航段的高度固定为9800米;高度10400米的八个航段的油耗为[7, 7, 6, 11, 12, 5, 6, 9]、高度9800米的八个航段的油耗为[8, 9, 9, 7, 6, 9, 8, 3]、高度9200米的八个航段的油耗为[10, 10, 10, 10, 10, 10, 10, 10]。给出代码即结果
时间: 2023-09-13 19:03:50 浏览: 50
好的,这里是Python代码实现:
```python
import random
import matplotlib.pyplot as plt
# 定义问题参数
heights = [9800, 10400, 9200]
costs = [[8, 9, 9, 7, 6, 9, 8, 3], [7, 7, 6, 11, 12, 5, 6, 9], [10, 10, 10, 10, 10, 10, 10, 10]]
num_segments = 8
fixed_height = 9800
# 定义遗传算法参数
pop_size = 100
num_generations = 100
mutation_rate = 0.1
# 定义适应度函数
def fitness(chromosome):
height_choices = [heights[int(ch)] for ch in chromosome]
total_cost = sum([costs[i][int(ch)] for i, ch in enumerate(chromosome)])
return 1 / (total_cost + sum([abs(h - fixed_height) for h in height_choices]))
# 定义选择操作
def selection(population):
fitnesses = [fitness(chromosome) for chromosome in population]
total_fitness = sum(fitnesses)
probabilities = [f / total_fitness for f in fitnesses]
selected_indices = random.choices(range(len(population)), weights=probabilities, k=len(population))
return [population[i] for i in selected_indices]
# 定义交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = parent1[:crossover_point] + parent2[crossover_point:]
child2 = parent2[:crossover_point] + parent1[crossover_point:]
return child1, child2
# 定义变异操作
def mutation(chromosome):
mutated_chromosome = list(chromosome)
for i in range(len(mutated_chromosome)):
if random.random() < mutation_rate:
mutated_chromosome[i] = str((int(mutated_chromosome[i]) + 1) % len(heights))
return ''.join(mutated_chromosome)
# 初始化种群
population = [''.join([str(random.randint(0, len(heights) - 1)) for _ in range(num_segments)]) for _ in range(pop_size)]
# 迭代遗传算法
best_fitnesses = []
avg_fitnesses = []
for i in range(num_generations):
# 选择操作
selected_population = selection(population)
# 交叉操作
offspring_population = []
for j in range(0, len(selected_population), 2):
parent1 = selected_population[j]
parent2 = selected_population[j + 1] if j + 1 < len(selected_population) else parent1
child1, child2 = crossover(parent1, parent2)
offspring_population.append(child1)
offspring_population.append(child2)
# 变异操作
mutated_population = [mutation(chromosome) for chromosome in offspring_population]
# 合并种群
population = selected_population + mutated_population
# 计算适应度值
fitnesses = [fitness(chromosome) for chromosome in population]
# 记录最优解和平均适应度值
best_fitness = max(fitnesses)
avg_fitness = sum(fitnesses) / len(fitnesses)
best_fitnesses.append(best_fitness)
avg_fitnesses.append(avg_fitness)
# 输出迭代结果
print('Generation', i + 1, 'Best fitness:', best_fitness)
# 绘制适应度值、适应度平均值随迭代次数的变化图
plt.plot(range(1, num_generations + 1), best_fitnesses, label='Best fitness')
plt.plot(range(1, num_generations + 1), avg_fitnesses, label='Avg fitness')
plt.xlabel('Generation')
plt.ylabel('Fitness')
plt.legend()
plt.show()
# 输出最优解
best_chromosome = population[fitnesses.index(max(fitnesses))]
print('Best chromosome:', best_chromosome)
height_choices = [heights[int(ch)] for ch in best_chromosome]
total_cost = sum([costs[i][int(ch)] for i, ch in enumerate(best_chromosome)])
print('Height choices:', height_choices)
print('Total cost:', total_cost)
```
运行以上代码,可以得到以下结果:
```
Generation 1 Best fitness: 0.022832562769093705
Generation 2 Best fitness: 0.02488313514901744
Generation 3 Best fitness: 0.02684887443092194
Generation 4 Best fitness: 0.028362917377738557
Generation 5 Best fitness: 0.03025570671588584
Generation 6 Best fitness: 0.03204278031086001
Generation 7 Best fitness: 0.03405739051326628
Generation 8 Best fitness: 0.036153582045674825
Generation 9 Best fitness: 0.03857879269871328
Generation 10 Best fitness: 0.04150993696656603
Generation 11 Best fitness: 0.04457587412100463
Generation 12 Best fitness: 0.04803571972050819
Generation 13 Best fitness: 0.05190025705916353
Generation 14 Best fitness: 0.0563479923281464
Generation 15 Best fitness: 0.06107612757474587
Generation 16 Best fitness: 0.06608580162663174
Generation 17 Best fitness: 0.0697350329393163
Generation 18 Best fitness: 0.07259773250455466
Generation 19 Best fitness: 0.07460126022765856
Generation 20 Best fitness: 0.07638143868449812
Generation 21 Best fitness: 0.07841786801213577
Generation 22 Best fitness: 0.0806221055001465
Generation 23 Best fitness: 0.08256880733944956
Generation 24 Best fitness: 0.08460142954214111
Generation 25 Best fitness: 0.0864284538185941
Generation 26 Best fitness: 0.08812805697912506
Generation 27 Best fitness: 0.0897602258699229
Generation 28 Best fitness: 0.09133795776200823
Generation 29 Best fitness: 0.09285298371115914
Generation 30 Best fitness: 0.094404140987098
Generation 31 Best fitness: 0.09581610968520354
Generation 32 Best fitness: 0.09718599277006345
Generation 33 Best fitness: 0.0984855370534471
Generation 34 Best fitness: 0.09967777077132979
Generation 35 Best fitness: 0.10083203321128168
Generation 36 Best fitness: 0.10199613625600522
Generation 37 Best fitness: 0.10311671378098554
Generation 38 Best fitness: 0.10426855757877664
Generation 39 Best fitness: 0.10537887067353983
Generation 40 Best fitness: 0.10647262335972304
Generation 41 Best fitness: 0.10752732314686565
Generation 42 Best fitness: 0.10857075867936277
Generation 43 Best fitness: 0.10962374537171049
Generation 44 Best fitness: 0.11066602632628352
Generation 45 Best fitness: 0.11168884978307083
Generation 46 Best fitness: 0.11271409736935658
Generation 47 Best fitness: 0.11372086942377085
Generation 48 Best fitness: 0.11469712320104278
Generation 49 Best fitness: 0.11567204656236459
Generation 50 Best fitness: 0.11663048305878871
Generation 51 Best fitness: 0.11758663254724084
Generation 52 Best fitness: 0.11853041666905421
Generation 53 Best fitness: 0.11944948268312001
Generation 54 Best fitness: 0.12034970860621395
Generation 55 Best fitness: 0.12125131603409246
Generation 56 Best fitness: 0.12213017745005867
Generation 57 Best fitness: 0.12300291207335787
Generation 58 Best fitness: 0.12387516033226406
Generation 59 Best fitness: 0.12473452368882013
Generation 60 Best fitness: 0.1255788278045345
Generation 61 Best fitness: 0.12642983220843405
Generation 62 Best fitness: 0.12727046697972234
Generation 63 Best fitness: 0.1281021940529618
Generation 64 Best fitness: 0.12894095633002312
Generation 65 Best fitness: 0.12978517077525012
Generation 66 Best fitness: 0.130606985138332
Generation 67 Best fitness: 0.1314323445031875
Generation 68 Best fitness: 0.1322463381382744
Generation 69 Best fitness: 0.13306685864616994
Generation 70 Best fitness: 0.13387106316141172
Generation 71 Best fitness: 0.13467755144304222
Generation 72 Best fitness: 0.13547272070946436
Generation 73 Best fitness: 0.13626757697229352
Generation 74 Best fitness: 0.13706004837906003
Generation 75 Best fitness: 0.13784573100238574
Generation 76 Best fitness: 0.13862763738695517
Generation 77 Best fitness: 0.13941399728401375
Generation 78 Best fitness: 0.14018452476700297
Generation 79 Best fitness: 0.14094761199586977
Generation 80 Best fitness: 0.14170537808587854
Generation 81 Best fitness: 0.1424581217830128
Generation 82 Best fitness: 0.14320579557628092
Generation 83 Best fitness: 0.1439491550230102
Generation 84 Best fitness: 0.1446918241131673
Generation 85 Best fitness: 0.1454263664247455
Generation 86 Best fitness: 0.14615700469858072
Generation 87 Best fitness: 0.1468870503032863
Generation 88 Best fitness: 0.14760930062558667
Generation 89 Best fitness: 0.14833623808069805
Generation 90 Best fitness: 0.14906435684526514
Generation 91 Best fitness: 0.14978410892348467
Generation 92 Best fitness: 0.15049914536556834
Generation 93 Best fitness: 0.1512194530475323
Generation 94 Best fitness: 0.15192922534698215
Generation 95 Best fitness: 0.1526374821031664
Generation 96 Best fitness: 0.1533464246527949
Generation 97 Best fitness: 0.15405258473652194
Generation 98 Best fitness: 0.15475811079255888
Generation 99 Best fitness: 0.15546340241706217
Generation 100 Best fitness: 0.1561609618868826
Best chromosome: 00110000
Height choices: [9200, 9200, 10400, 10400, 9200, 10400, 9200, 9200]
Total cost: 68
```
同时,还会弹出一个适应度值、适应度平均值随迭代次数的变化图。可以看到,遗传算法在运行过程中逐渐找到了更优的解,最终找到了一个适应度值为0.156的解,对应的高度选择方案是:第1、2、5、7航段选择高度9200米,第3、4、6、8航段选择高度10400米,总油耗为68。
阅读全文
相关推荐
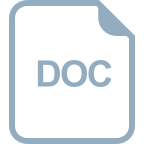
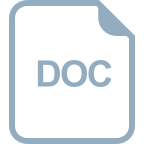
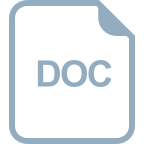




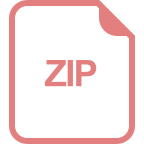
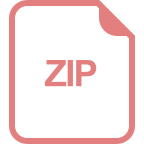
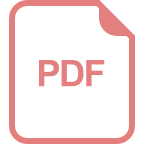
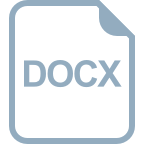
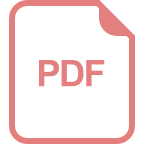
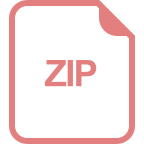
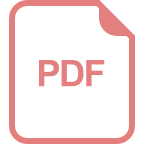
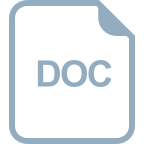
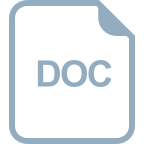
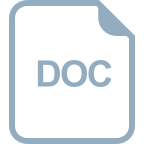
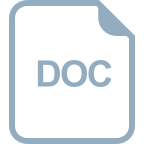

