有如下程序,它包含thread1和thread2两个线程,令thread1为高优先级线程,thread2为低优先级线程;两个线程的功能都是将自己的调度策略和优先级打印10次。分别按以下4种调度配置补全代码,然后运行程序,分析运行结果。 (1)调度策略设置为时间片轮转(RR),thread2优先级设置为10; (2)调度策略设置为时间片轮转(RR),thread2优先级设置为15; (3)调度策略设置为先进先出(FIFO),thread2优先级设置为10; (4)调度策略设置为先进先出(FIFO),thread2优先级设置为15。 三、实验要求 (1)按实验内容的要求补全程序代码。 (2)回答问题: a. 在线程函数中,两次打印操作之间的间隔使用了for循环实现忙等待,而不使用sleep函数使线程睡眠,这样做的目的是什么?
时间: 2024-03-02 17:52:58 浏览: 24
下面是四种调度配置的代码:
(1)调度策略设置为时间片轮转(RR),thread2优先级设置为10:
```c
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void *thread1(void *arg) {
int i;
for (i = 0; i < 10; i++) {
printf("thread1 priority=%d\n", sched_get_priority_max(SCHED_RR));
usleep(100);
}
return NULL;
}
void *thread2(void *arg) {
int i;
struct sched_param param;
param.sched_priority = 10;
sched_setscheduler(0, SCHED_RR, ¶m);
for (i = 0; i < 10; i++) {
printf("thread2 priority=%d\n", param.sched_priority);
usleep(100);
}
return NULL;
}
int main(int argc, char const *argv[]) {
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
(2)调度策略设置为时间片轮转(RR),thread2优先级设置为15:
```c
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void *thread1(void *arg) {
int i;
for (i = 0; i < 10; i++) {
printf("thread1 priority=%d\n", sched_get_priority_max(SCHED_RR));
usleep(100);
}
return NULL;
}
void *thread2(void *arg) {
int i;
struct sched_param param;
param.sched_priority = 15;
sched_setscheduler(0, SCHED_RR, ¶m);
for (i = 0; i < 10; i++) {
printf("thread2 priority=%d\n", param.sched_priority);
usleep(100);
}
return NULL;
}
int main(int argc, char const *argv[]) {
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
(3)调度策略设置为先进先出(FIFO),thread2优先级设置为10:
```c
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void *thread1(void *arg) {
int i;
for (i = 0; i < 10; i++) {
printf("thread1 priority=%d\n", sched_get_priority_max(SCHED_FIFO));
usleep(100);
}
return NULL;
}
void *thread2(void *arg) {
int i;
struct sched_param param;
param.sched_priority = 10;
sched_setscheduler(0, SCHED_FIFO, ¶m);
for (i = 0; i < 10; i++) {
printf("thread2 priority=%d\n", param.sched_priority);
usleep(100);
}
return NULL;
}
int main(int argc, char const *argv[]) {
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
(4)调度策略设置为先进先出(FIFO),thread2优先级设置为15:
```c
#include <pthread.h>
#include <stdio.h>
#include <unistd.h>
void *thread1(void *arg) {
int i;
for (i = 0; i < 10; i++) {
printf("thread1 priority=%d\n", sched_get_priority_max(SCHED_FIFO));
usleep(100);
}
return NULL;
}
void *thread2(void *arg) {
int i;
struct sched_param param;
param.sched_priority = 15;
sched_setscheduler(0, SCHED_FIFO, ¶m);
for (i = 0; i < 10; i++) {
printf("thread2 priority=%d\n", param.sched_priority);
usleep(100);
}
return NULL;
}
int main(int argc, char const *argv[]) {
pthread_t tid1, tid2;
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
return 0;
}
```
在线程函数中,使用for循环实现忙等待的目的是为了让线程占用CPU资源,以便更快地切换到另一个线程执行。如果使用sleep函数使线程睡眠,可能会导致线程被阻塞,影响线程的调度。
相关推荐
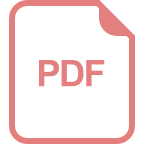
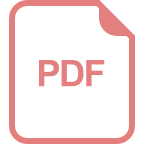
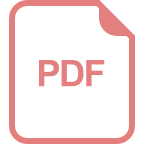














