用c语言编程计算某年某月有几天
时间: 2024-11-18 08:27:21 浏览: 1
在 C 语言中,计算某年某月有多少天通常需要考虑一些特殊情况,比如闰年的判断和二月份的天数。下面是一个简单的函数示例,用于计算非闰年普通月份的天数,以及闰年二月的天数:
```c
#include <stdio.h>
int is_leap_year(int year) {
// 判断是否为闰年规则:能被4整除但不能被100整除,或者能被400整除
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
int days_in_month(int month, int year) {
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12: // 非闰年,每个月31天
return 31;
case 2: // 闰年和平年对2月天数的影响
if (is_leap_year(year))
return 29;
else
return 28;
case 4:
case 6:
case 9:
case 11: // 其他月份都是30天
return 30;
default:
printf("Invalid month number.\n");
return -1; // 无效的月份,返回错误值
}
}
int main() {
int month, year;
printf("Enter the month and year (e.g., 2 2020): ");
scanf("%d %d", &month, &year);
int days = days_in_month(month, year);
if (days != -1)
printf("Month %d of %d has %d days.\n", month, year, days);
else
printf("Failed to calculate the number of days.\n");
return 0;
}
```
阅读全文
相关推荐
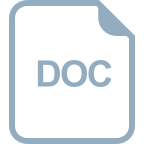
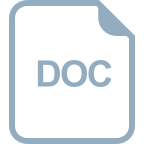
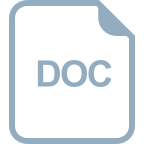





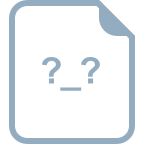
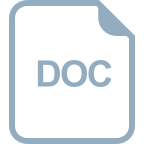
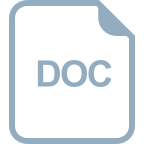
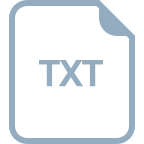
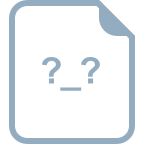
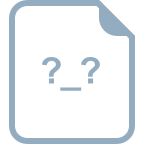



