最小二乘法多项式拟合代码
时间: 2023-11-12 21:00:34 浏览: 47
好的,以下是最小二乘法多项式拟合的 Python 代码:
```python
import numpy as np
def polyfit(x, y, degree):
results = {}
coeffs = np.polyfit(x, y, degree)
# Polynomial Coefficients
results['polynomial'] = coeffs.tolist()
# r-squared
p = np.poly1d(coeffs)
yhat = p(x)
ybar = np.sum(y)/len(y)
ssreg = np.sum((yhat-ybar)**2)
sstot = np.sum((y - ybar)**2)
results['r_squared'] = ssreg / sstot
return results
```
其中,x 和 y 分别为输入的数据点的横纵坐标,degree 为拟合多项式的次数。函数返回一个字典,包含拟合多项式的系数和 R-squared 值。
相关问题
matlab最小二乘法多项式拟合代码
好的,以下是matlab最小二乘法多项式拟合代码:
```matlab
% x和y分别为自变量和因变量
% n为多项式次数
function [p, S] = polyfit_least_squares(x, y, n)
X = zeros(length(x), n+1);
for i = 1:n+1
X(:,i) = x.^(n+1-i);
end
[Q,R] = qr(X);
p = R\(Q'*y);
S = norm(y-X*p)^2;
end
```
其中,`x`和`y`分别为自变量和因变量,`n`为多项式次数。函数返回值`p`为拟合多项式的系数,`S`为残差平方和。
最小二乘法多项式拟合python代码
以下是使用最小二乘法进行多项式拟合的Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成随机数据
x = np.linspace(-np.pi, np.pi, 50)
y = np.sin(x) + np.random.rand(50) * 0.2
# 多项式拟合
p = np.polyfit(x, y, 5) # 用5次多项式拟合
# 绘制图像
plt.plot(x, y, 'o')
plt.plot(x, np.polyval(p, x), 'r')
plt.show()
```
在上面的示例中,我们首先生成了50个带有噪声的正弦函数数据。然后使用`np.polyfit()`函数进行多项式拟合,拟合次数为5。接着,使用`np.polyval()`函数计算出拟合的多项式函数的值,并将其绘制在图像上。
注意:最小二乘法拟合的多项式次数越高,越容易出现过拟合现象。因此,在实际应用中需要谨慎选择拟合次数。
相关推荐
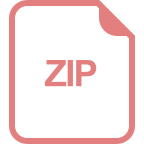












