linux 下std::thread多线程如何减少CPU占用率
时间: 2023-08-18 09:04:53 浏览: 56
在Linux下,可以使用一些方法来减少std::thread多线程的CPU占用率:
1. 使用sleep或者wait方法:在多线程中,可以在每个线程的循环中添加一个sleep或者wait方法,使线程在执行完当前任务后进入休眠状态,从而减少CPU的占用率。可以根据实际需求设置合适的休眠时间。
2. 使用互斥锁(mutex)和条件变量(condition variable):通过使用互斥锁和条件变量,可以实现线程的同步和等待。当线程没有任务可执行时,可以让线程等待条件变量的触发,从而减少CPU的占用率。
3. 使用线程池:创建一个线程池,将任务分配给线程池中的线程执行,线程在执行完任务后可以继续等待新的任务而不是退出,从而减少线程的频繁创建和销毁,降低CPU的占用率。
4. 使用线程优先级:可以通过设置线程的优先级来控制线程的执行顺序和占用CPU的时间。将一些低优先级的线程设置为较低的优先级,从而减少它们对CPU的占用。
5. 使用CPU亲和性(CPU affinity):可以将线程绑定到特定的CPU核心上,从而控制线程在特定CPU上执行,减少线程的切换和竞争,降低CPU的占用率。
这些方法可以根据具体的需求和场景来选择和组合使用,以达到减少CPU占用率的目的。
相关问题
linux 下C++程序std::thread多线程如何减少CPU占用率,请分别举例说明
当使用C++程序中的std::thread创建多线程时,可以采取以下方法来降低CPU占用率:
1. 调整线程的睡眠时间:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
void threadFunc() {
while (true) {
// 在线程函数中加入适当的睡眠时间,降低CPU占用率
std::this_thread::sleep_for(std::chrono::milliseconds(10));
// 其他操作
}
}
int main() {
std::thread t(threadFunc);
t.join();
return 0;
}
```
在上述示例中,线程函数`threadFunc`在每次循环时都会睡眠10毫秒,从而降低了CPU占用率。根据实际需求,可以调整睡眠时间。
2. 使用互斥锁和条件变量:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool isReady = false;
void threadFunc() {
std::unique_lock<std::mutex> lock(mtx);
while (true) {
// 等待条件变量
cv.wait(lock, []{ return isReady; });
// 其他操作
}
}
int main() {
std::thread t(threadFunc);
// 模拟主线程设置条件变量的状态
std::this_thread::sleep_for(std::chrono::seconds(1));
{
std::lock_guard<std::mutex> lock(mtx);
isReady = true;
}
cv.notify_one();
t.join();
return 0;
}
```
在上述示例中,线程函数`threadFunc`会等待条件变量`isReady`为true时才执行其他操作。通过使用互斥锁和条件变量,可以避免线程不必要地竞争资源,从而降低CPU占用率。
这些是常见的方法,具体的应用场景可能需要根据实际需求进行调整和扩展。同时,还可以考虑使用线程池等更高级的技术来管理多线程,以进一步减少CPU占用率。
linux 下C++程序std::thread多线程如何调整调度策略和时间片减少CPU占用率,请分别举例说明
在 Linux 下,可以使用以下方法来调整 std::thread 多线程的调度策略和时间片,从而减少 CPU 占用率:
1. 调整调度策略:可以使用 sched_setscheduler() 函数来修改线程的调度策略。常见的调度策略有 SCHED_OTHER、SCHED_FIFO 和 SCHED_RR。其中,SCHED_OTHER 是默认的调度策略,SCHED_FIFO 和 SCHED_RR 是实时调度策略。实时调度策略可以优先于默认的调度策略进行调度,从而降低 CPU 占用率。
示例代码如下:
```cpp
#include <pthread.h>
#include <iostream>
void* threadFunc(void* arg) {
// 线程函数的实现
}
int main() {
// 创建线程
pthread_t thread;
pthread_create(&thread, NULL, threadFunc, NULL);
// 设置线程的调度策略为 SCHED_FIFO
struct sched_param param;
param.sched_priority = 1;
pthread_setschedparam(thread, SCHED_FIFO, ¶m);
// 等待线程结束
pthread_join(thread, NULL);
return 0;
}
```
在上面的示例中,通过 pthread_setschedparam() 函数将线程的调度策略设置为 SCHED_FIFO,并指定优先级为 1。这样,线程将以实时调度的方式运行。
2. 减少时间片:可以使用 sched_yield() 函数来主动放弃当前线程的时间片,从而降低 CPU 占用率。
示例代码如下:
```cpp
#include <thread>
#include <iostream>
#include <chrono>
void threadFunc() {
// 线程函数的实现
}
int main() {
// 创建线程
std::thread thread(threadFunc);
// 主动放弃时间片,降低 CPU 占用率
while (true) {
std::this_thread::sleep_for(std::chrono::milliseconds(10));
std::this_thread::yield();
}
// 等待线程结束
thread.join();
return 0;
}
```
在上面的示例中,通过在主线程中使用 std::this_thread::yield() 函数,主动放弃时间片,从而降低 CPU 占用率。
以上是两种常见的方法来调整 std::thread 多线程的调度策略和时间片,以减少 CPU 占用率。
相关推荐
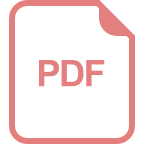
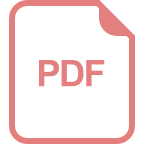
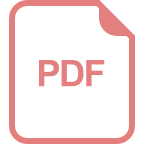












