C语音十进制转换成二进制代码
时间: 2024-09-29 13:05:36 浏览: 48
在C语言中,将十进制整数转换为二进制字符串,可以使用位运算和数组来实现。下面是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// Function to convert decimal number to binary string
void decToBinary(int num, char binary[50]) {
int i = 0;
while (num > 0) {
binary[i] = num % 2 + '0'; // Convert remainder to ASCII and store in string
num = num / 2; // Shift the bits right
i++;
}
binary[i] = '\0'; // Add null terminator
// Reverse the string since we append from right to left
reverse(binary, 0, strlen(binary) - 1);
}
// Helper function for reversing a string
void reverse(char *str, int start, int end) {
while (start < end) {
char temp = str[start];
str[start] = str[end];
str[end] = temp;
start++;
end--;
}
}
int main() {
int decimalNum = 42; // Your input decimal number
char binary[50]; // Buffer to store the binary result
decToBinary(decimalNum, binary);
printf("Decimal number %d is in binary: %s\n", decimalNum, binary);
return 0;
}
```
在这个例子中,`decToBinary`函数会把输入的十进制数字转换为二进制字符串,并存储到`binary`数组中。`reverse`函数用于反转字符串,因为在填充数组时是从低位向高位添加的。
阅读全文
相关推荐











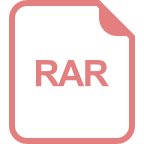

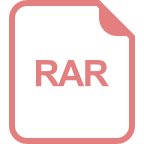



