用javascript在网页实现推箱子游戏,至少包含1个箱子和洞,玩家能上下左右自由移动。地图16*16,洞在四个角,人在中心,箱子在人的上下左右四个相邻格子上。
时间: 2024-03-11 13:49:55 浏览: 126
好的,我可以为您提供一些代码示例来实现推箱子游戏。
首先,在 HTML 中创建一个 div 元素作为游戏区域,并使用 CSS 设置其样式和大小:
```html
<!DOCTYPE html>
<html>
<head>
<title>推箱子游戏</title>
<style>
#game-board {
width: 480px;
height: 480px;
display: grid;
grid-template-columns: repeat(16, 1fr);
grid-template-rows: repeat(16, 1fr);
}
.cell {
border: 1px solid #ccc;
box-sizing: border-box;
}
.hole {
background-color: #333;
}
.player {
background-color: #f00;
}
.box {
background-color: #0f0;
}
</style>
</head>
<body>
<div id="game-board"></div>
<script>
// 在这里编写 JavaScript 代码
</script>
</body>
</html>
```
接下来,使用 JavaScript 创建地图,并在其中添加洞、玩家和箱子:
```javascript
const gameBoard = document.getElementById('game-board');
const map = [
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
];
for (let i = 0; i < 16; i++) {
for (let j = 0; j < 16; j++) {
const cell = document.createElement('div');
cell.className = 'cell';
if (map[i][j] === 1) {
cell.className += ' wall';
}
if (i === 0 && j === 0 || i === 0 && j === 15 || i === 15 && j === 0 || i === 15 && j === 15) {
cell.className += ' hole';
}
if (i === 7 && j === 7) {
cell.className += ' player';
}
if (i === 6 && j === 7 || i === 8 && j === 7 || i === 7 && j === 6 || i === 7 && j === 8) {
cell.className += ' box';
}
gameBoard.appendChild(cell);
}
}
```
最后,监听键盘事件,控制玩家移动和箱子推动:
```javascript
const player = document.querySelector('.player');
const boxes = document.querySelectorAll('.box');
document.addEventListener('keydown', (event) => {
let x = 0;
let y = 0;
if (event.key === 'ArrowUp') {
y = -1;
} else if (event.key === 'ArrowDown') {
y = 1;
} else if (event.key === 'ArrowLeft') {
x = -1;
} else if (event.key === 'ArrowRight') {
x = 1;
}
const nextCell = document.querySelector(`.cell[data-x="${player.dataset.x * 1 + x}"][data-y="${player.dataset.y * 1 + y}"]`);
if (nextCell && !nextCell.classList.contains('wall') && !nextCell.classList.contains('box')) {
player.dataset.x = player.dataset.x * 1 + x;
player.dataset.y = player.dataset.y * 1 + y;
player.style.gridRow = player.dataset.y * 1 + 1;
player.style.gridColumn = player.dataset.x * 1 + 1;
} else if (nextCell && nextCell.classList.contains('box')) {
const nextBoxCell = document.querySelector(`.cell[data-x="${nextCell.dataset.x * 1 + x}"][data-y="${nextCell.dataset.y * 1 + y}"]`);
if (nextBoxCell && !nextBoxCell.classList.contains('wall') && !nextBoxCell.classList.contains('box')) {
nextCell.classList.remove('box');
nextBoxCell.classList.add('box');
nextBoxCell.dataset.box = true;
nextCell.dataset.box = false;
player.dataset.x = player.dataset.x * 1 + x;
player.dataset.y = player.dataset.y * 1 + y;
player.style.gridRow = player.dataset.y * 1 + 1;
player.style.gridColumn = player.dataset.x * 1 + 1;
}
}
boxes.forEach((box) => {
if (box.dataset.box === 'true') {
box.dataset.x = box.parentNode.dataset.x;
box.dataset.y = box.parentNode.dataset.y;
}
});
});
```
这样,一个简单的推箱子游戏就完成了。您可以根据需要对代码进行修改和优化。
阅读全文
相关推荐
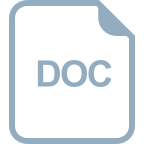
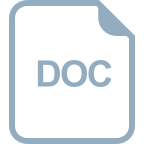
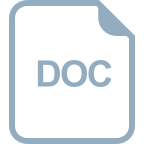
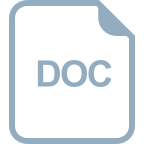
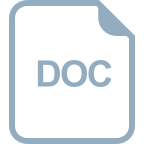
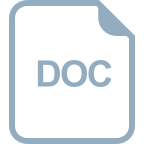

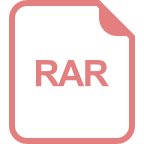
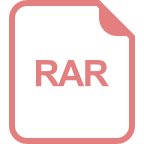
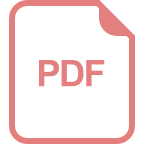
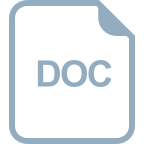


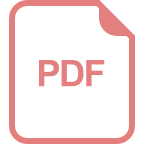
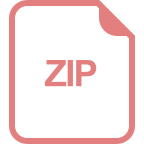
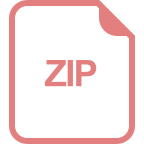
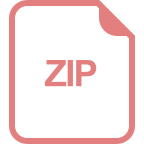
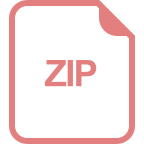
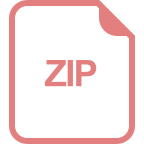