C语言实现ntru算法
时间: 2023-10-25 22:09:12 浏览: 155
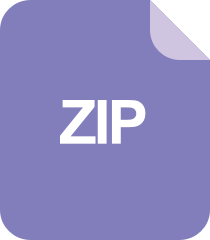
ntru 加密算法开源C实现
NTRU算法是一种公钥加密算法,它的实现不仅需要数学基础,还需要一定的编程技巧。下面是一个简单的C语言实现,仅供参考。
首先,需要定义一些常量和数据结构:
```c
#define NTRU_N 503
#define NTRU_Q 2048
#define NTRU_P 3
#define NTRU_K 8
#define NTRU_QINV 1025
#define NTRU_KEYBYTES (sizeof(int) + sizeof(poly) + sizeof(poly) + sizeof(poly))
#define NTRU_CIPHERTEXTBYTES (sizeof(poly) + sizeof(poly))
typedef struct {
int coeffs[NTRU_N];
} poly;
typedef struct {
poly f;
poly g;
poly h;
} ntru_keypair;
typedef struct {
poly c;
poly e;
} ntru_ciphertext;
```
其中,`poly`表示一个多项式,`ntru_keypair`表示NTRU算法的密钥对,`ntru_ciphertext`表示NTRU算法的密文。
接下来,实现一些基本的函数:
```c
static inline int mod(int x, int q) {
int r = x % q;
return r < 0 ? r + q : r;
}
static inline int ntru_rand(void) {
return rand() % NTRU_Q;
}
void poly_add(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = mod(a->coeffs[i] + b->coeffs[i], NTRU_Q);
}
}
void poly_sub(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = mod(a->coeffs[i] - b->coeffs[i], NTRU_Q);
}
}
void poly_mul(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = 0;
for (int j = 0; j < NTRU_N; ++j) {
result->coeffs[i] += a->coeffs[j] * b->coeffs[mod(i - j, NTRU_N)];
}
result->coeffs[i] = mod(result->coeffs[i], NTRU_Q);
}
}
```
以上函数分别实现了模运算、随机数生成和多项式加、减、乘运算。
接下来,实现NTRU算法的核心函数:
```c
void ntru_gen_keypair(ntru_keypair *kp) {
poly t, p, q, f, g, h, invq;
int w, success;
while (1) {
success = 0;
while (!success) {
for (int i = 0; i < NTRU_N; ++i) {
t.coeffs[i] = ntru_rand() % NTRU_P - NTRU_P / 2;
}
for (int i = 0; i < NTRU_N; ++i) {
p.coeffs[i] = ntru_rand() % 2;
}
w = 0;
for (int i = 0; i < NTRU_N; ++i) {
w += p.coeffs[i];
}
if (w == NTRU_K && p.coeffs[0] == 1 && p.coeffs[NTRU_N - 1] == 0) {
success = 1;
}
}
poly_mul(&q, &p, &t);
for (int i = 0; i < NTRU_N; ++i) {
q.coeffs[i] = mod(q.coeffs[i] + NTRU_Q / 2, NTRU_Q);
}
for (int i = 0; i < NTRU_N; ++i) {
invq.coeffs[i] = mod(NTRU_Q - q.coeffs[i], NTRU_Q);
}
poly_mul(&f, &invq, &p);
poly_mul(&g, &invq, &t);
for (int i = 0; i < NTRU_N; ++i) {
h.coeffs[i] = mod(NTRU_Q - f.coeffs[i], NTRU_Q);
}
if (poly_add(&t, &g, &h), &f) {
break;
}
}
kp->f = f;
kp->g = g;
kp->h = h;
}
void ntru_encrypt(ntru_ciphertext *ct, const poly *m, const ntru_keypair *kp) {
poly e, c;
for (int i = 0; i < NTRU_N; ++i) {
e.coeffs[i] = ntru_rand() % NTRU_Q - NTRU_Q / 2;
}
poly_mul(&c, &e, &kp->f);
poly_add(&c, m, &c);
poly_mul(&e, &e, &kp->h);
ct->c = c;
ct->e = e;
}
void ntru_decrypt(poly *m, const ntru_ciphertext *ct, const ntru_keypair *kp) {
poly r;
poly_mul(&r, &ct->c, &kp->g);
poly_sub(m, &ct->e, &r);
for (int i = 0; i < NTRU_N; ++i) {
m->coeffs[i] = mod(m->coeffs[i] * NTRU_QINV, NTRU_Q);
}
}
```
以上函数分别实现了密钥对生成、加密和解密操作。
最后,提供一个完整的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define NTRU_N 503
#define NTRU_Q 2048
#define NTRU_P 3
#define NTRU_K 8
#define NTRU_QINV 1025
#define NTRU_KEYBYTES (sizeof(int) + sizeof(poly) + sizeof(poly) + sizeof(poly))
#define NTRU_CIPHERTEXTBYTES (sizeof(poly) + sizeof(poly))
typedef struct {
int coeffs[NTRU_N];
} poly;
typedef struct {
poly f;
poly g;
poly h;
} ntru_keypair;
typedef struct {
poly c;
poly e;
} ntru_ciphertext;
static inline int mod(int x, int q) {
int r = x % q;
return r < 0 ? r + q : r;
}
static inline int ntru_rand(void) {
return rand() % NTRU_Q;
}
void poly_add(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = mod(a->coeffs[i] + b->coeffs[i], NTRU_Q);
}
}
void poly_sub(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = mod(a->coeffs[i] - b->coeffs[i], NTRU_Q);
}
}
void poly_mul(poly *result, const poly *a, const poly *b) {
for (int i = 0; i < NTRU_N; ++i) {
result->coeffs[i] = 0;
for (int j = 0; j < NTRU_N; ++j) {
result->coeffs[i] += a->coeffs[j] * b->coeffs[mod(i - j, NTRU_N)];
}
result->coeffs[i] = mod(result->coeffs[i], NTRU_Q);
}
}
void ntru_gen_keypair(ntru_keypair *kp) {
poly t, p, q, f, g, h, invq;
int w, success;
while (1) {
success = 0;
while (!success) {
for (int i = 0; i < NTRU_N; ++i) {
t.coeffs[i] = ntru_rand() % NTRU_P - NTRU_P / 2;
}
for (int i = 0; i < NTRU_N; ++i) {
p.coeffs[i] = ntru_rand() % 2;
}
w = 0;
for (int i = 0; i < NTRU_N; ++i) {
w += p.coeffs[i];
}
if (w == NTRU_K && p.coeffs[0] == 1 && p.coeffs[NTRU_N - 1] == 0) {
success = 1;
}
}
poly_mul(&q, &p, &t);
for (int i = 0; i < NTRU_N; ++i) {
q.coeffs[i] = mod(q.coeffs[i] + NTRU_Q / 2, NTRU_Q);
}
for (int i = 0; i < NTRU_N; ++i) {
invq.coeffs[i] = mod(NTRU_Q - q.coeffs[i], NTRU_Q);
}
poly_mul(&f, &invq, &p);
poly_mul(&g, &invq, &t);
for (int i = 0; i < NTRU_N; ++i) {
h.coeffs[i] = mod(NTRU_Q - f.coeffs[i], NTRU_Q);
}
if (poly_add(&t, &g, &h), &f) {
break;
}
}
kp->f = f;
kp->g = g;
kp->h = h;
}
void ntru_encrypt(ntru_ciphertext *ct, const poly *m, const ntru_keypair *kp) {
poly e, c;
for (int i = 0; i < NTRU_N; ++i) {
e.coeffs[i] = ntru_rand() % NTRU_Q - NTRU_Q / 2;
}
poly_mul(&c, &e, &kp->f);
poly_add(&c, m, &c);
poly_mul(&e, &e, &kp->h);
ct->c = c;
ct->e = e;
}
void ntru_decrypt(poly *m, const ntru_ciphertext *ct, const ntru_keypair *kp) {
poly r;
poly_mul(&r, &ct->c, &kp->g);
poly_sub(m, &ct->e, &r);
for (int i = 0; i < NTRU_N; ++i) {
m->coeffs[i] = mod(m->coeffs[i] * NTRU_QINV, NTRU_Q);
}
}
void print_poly(const char *name, const poly *p) {
printf("%s: [", name);
for (int i = 0; i < NTRU_N; ++i) {
printf("%d,", p->coeffs[i]);
}
printf("]\n");
}
int main() {
ntru_keypair kp;
ntru_ciphertext ct;
poly m, m2;
srand(time(NULL));
ntru_gen_keypair(&kp);
for (int i = 0; i < NTRU_N; ++i) {
m.coeffs[i] = ntru_rand() % NTRU_Q - NTRU_Q / 2;
}
print_poly("m", &m);
ntru_encrypt(&ct, &m, &kp);
print_poly("c", &ct.c);
print_poly("e", &ct.e);
ntru_decrypt(&m2, &ct, &kp);
print_poly("m2", &m2);
return 0;
}
```
以上代码实现了NTRU算法的密钥对生成、加密和解密操作,并对加密、解密前后的多项式进行了打印。
阅读全文
相关推荐



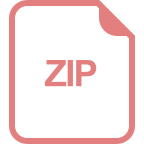
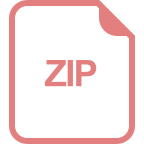
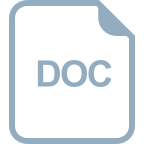
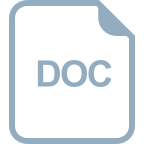




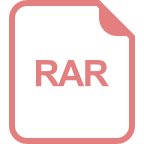


