stm32通过摄像头传回的物体坐标进行判断,使小车追上物体并停在物体前方使用pid算法,stm32代码
时间: 2024-02-24 14:55:03 浏览: 20
以下是基于STM32的摄像头物体追踪和PID控制的示例代码:
```c
#include "stm32f10x.h"
#include "camera.h"
// 定义PID控制参数
#define KP 0.5
#define KI 0.2
#define KD 0.1
// 定义小车状态
#define CAR_STOP 0
#define CAR_RUN 1
// 定义小车电机控制引脚
#define MOTOR1_A_PIN GPIO_Pin_0
#define MOTOR1_B_PIN GPIO_Pin_1
#define MOTOR2_A_PIN GPIO_Pin_2
#define MOTOR2_B_PIN GPIO_Pin_3
// 定义小车电机控制GPIO端口
#define MOTOR_PORT GPIOB
// 定义小车状态
uint8_t carState = CAR_STOP;
// 定义PID控制器变量
float last_error = 0;
float integral = 0;
int main(void)
{
// 初始化摄像头
camera_init();
// 初始化小车电机控制GPIO
GPIO_InitTypeDef GPIO_InitStructure;
GPIO_InitStructure.GPIO_Pin = MOTOR1_A_PIN | MOTOR1_B_PIN | MOTOR2_A_PIN | MOTOR2_B_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(MOTOR_PORT, &GPIO_InitStructure);
// 循环执行
while(1)
{
// 读取摄像头数据
uint16_t x, y;
camera_read(&x, &y);
// 如果没有检测到物体,停止小车
if(x == 0 && y == 0)
{
carState = CAR_STOP;
GPIO_ResetBits(MOTOR_PORT, MOTOR1_A_PIN | MOTOR1_B_PIN | MOTOR2_A_PIN | MOTOR2_B_PIN);
continue;
}
// 计算物体距离中心的偏差
float error = x - 160;
// 计算PID控制器输出
float output = KP * error + KI * integral + KD * (error - last_error);
// 更新PID控制器变量
integral += error;
last_error = error;
// 根据输出控制小车电机
if(output > 0)
{
GPIO_SetBits(MOTOR_PORT, MOTOR1_A_PIN | MOTOR2_B_PIN);
GPIO_ResetBits(MOTOR_PORT, MOTOR1_B_PIN | MOTOR2_A_PIN);
}
else
{
GPIO_SetBits(MOTOR_PORT, MOTOR1_B_PIN | MOTOR2_A_PIN);
GPIO_ResetBits(MOTOR_PORT, MOTOR1_A_PIN | MOTOR2_B_PIN);
}
// 设置小车状态为运动
carState = CAR_RUN;
}
}
```
以上代码仅为示例,具体实现要根据具体的硬件和摄像头接口进行修改。
相关推荐
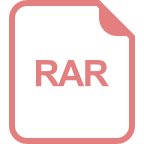
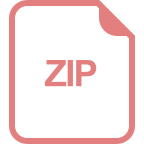
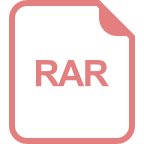














