使用c++写一个三角形旋转的程序
时间: 2023-12-03 11:02:43 浏览: 30
好的,以下是一个使用C++编写的三角形旋转程序:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.14159265358979323846;
struct Point {
double x, y;
Point(double x = 0, double y = 0) : x(x), y(y) {}
};
// 旋转函数,参数为旋转角度、旋转中心、需要旋转的点
Point rotate(double angle, Point center, Point p) {
double rad = angle * PI / 180.0; // 角度转弧度
double dx = p.x - center.x, dy = p.y - center.y;
double x = dx * cos(rad) - dy * sin(rad) + center.x;
double y = dx * sin(rad) + dy * cos(rad) + center.y;
return Point(x, y);
}
int main() {
// 初始化三角形的三个顶点
Point A(0, 0), B(2, 0), C(0, 2);
double angle;
cout << "请输入旋转角度(度):";
cin >> angle;
// 以三角形重心为旋转中心
Point center((A.x + B.x + C.x) / 3, (A.y + B.y + C.y) / 3);
// 分别对三个点进行旋转
A = rotate(angle, center, A);
B = rotate(angle, center, B);
C = rotate(angle, center, C);
// 输出旋转后的三个点
cout << "旋转后的三角形三个顶点坐标为:" << endl;
cout << "A(" << A.x << ", " << A.y << ")" << endl;
cout << "B(" << B.x << ", " << B.y << ")" << endl;
cout << "C(" << C.x << ", " << C.y << ")" << endl;
return 0;
}
```
这个程序会先输入旋转角度,然后以三角形重心为旋转中心,对三角形的三个顶点进行旋转,并输出旋转后的三个顶点坐标。可以自行修改三角形顶点的坐标来进行测试。
相关推荐
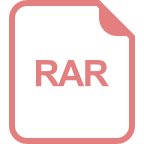
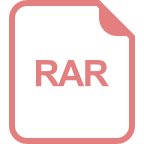














