C语言输出字符串中次数最多的单词及其出现次数
时间: 2023-11-28 18:46:31 浏览: 343
为了输出字符串中出现次数最多的单词及其出现次数,我们可以使用哈希表来存储每个单词出现的次数,然后按照出现次数从大到小排序,输出前5个单词及其出现次数。
以下是一个C语言的例子:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
#define MAX_WORDS 1000
int main() {
char str[MAX_WORDS];
int count[MAX_WORDS] = {0};
char words[MAX_WORDS][MAX_WORDS];
int i, j, k, len, max_count, word_count;
// 读入字符串
printf("请输入一个字符串:\n");
fgets(str, MAX_WORDS, stdin);
// 统计单词出现次数
len = strlen(str);
word_count = 0;
for (i = 0; i < len; i++) {
if (isalnum(str[i])) {
j = i;
while (isalnum(str[j])) {
j++;
}
strncpy(words[word_count], str + i, j - i);
words[word_count][j - i] = '\0';
word_count++;
i = j;
}
}
for (i = 0; i < word_count; i++) {
count[i] = 1;
for (j = i + 1; j < word_count; j++) {
if (strcmp(words[i], words[j]) == 0) {
count[i]++;
}
}
}
// 输出出现次数最多的前5个单词及其出现次数
printf("出现次数最多的前5个单词及其出现次数为:\n");
for (k = 0; k < 5; k++) {
max_count = 0;
for (i = 0; i < word_count; i++) {
if (count[i] > max_count) {
max_count = count[i];
j = i;
}
}
if (max_count == 0) {
break;
}
printf("%s: %d\n", words[j], max_count);
count[j] = 0;
}
return 0;
}
```
阅读全文
相关推荐








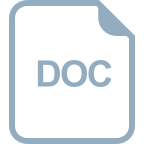









