如何检查元素在unordered set中
时间: 2024-09-21 14:12:12 浏览: 26
在C++标准库中,`std::unordered_set`是一个关联容器,用于存储唯一元素的无序集合。如果你想检查一个元素是否存在于`unordered_set`中,你可以使用它的成员函数`count()`。这个函数会返回指定值在集合中出现的次数。如果元素存在,`count()`会返回1;如果不存在,返回0。
下面是一个简单的例子:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4, 5};
int searchValue = 3;
if (mySet.count(searchValue) > 0) {
std::cout << "Element found in the set." << std::endl;
} else {
std::cout << "Element not found in the set." << std::endl;
}
return 0;
}
```
在这个示例中,`mySet.count(searchValue)`将检查3是否在集合中。如果结果大于0,表示找到,否则未找到。
相关问题
unorderedset 删除元素
要从unordered_set中删除元素,可以使用erase()函数。erase()函数有几种不同的用法,可以根据需要选择。以下是一些常见的用法:
1. 删除指定元素:可以使用erase()函数将指定元素从unordered_set中删除。例如,要删除元素x,可以使用unordered_set的erase(x)函数。
2. 删除一定范围内的元素:可以使用erase()函数删除一定范围内的元素。需要提供要删除的范围的起始和结束迭代器。例如,要删除从begin到end范围内的元素,可以使用unordered_set的erase(begin, end)函数。
3. 删除满足条件的元素:可以使用erase()函数结合lambda表达式来删除满足特定条件的元素。例如,要删除所有大于某个值的元素,可以使用unordered_set的erase()函数和lambda表达式来实现。
请注意,erase()函数会返回删除的元素的数量。如果要确定元素是否成功删除,可以检查返回的数量是否为0。
综上所述,要从unordered_set中删除元素,可以使用erase()函数,并根据需要选择不同的用法。<span class="em">1</span><span class="em">2</span>
#### 引用[.reference_title]
- *1* [unordered_set学习之删除操作clear,erase,swap,extract,merge](https://blog.csdn.net/chenyijun/article/details/119065095)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *2* [LeetCode判断字符串是否循环-Log:记录我的编码](https://download.csdn.net/download/weixin_38716081/19950130)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
unorderedset
unordered_set是C++标准库中的容器,它提供了一种高效的无序集合,其中的元素没有特定的顺序。它基于哈希表实现,因此查找、插入和删除操作的平均时间复杂度都是常数时间O(1)。
unordered_set中的元素必须是唯一的,重复的元素将被自动忽略。可以使用哈希函数来确定元素在内部存储中的位置,从而实现快速的查找和插入操作。
使用unordered_set时,你可以通过以下方式进行常见操作:
- 插入元素:使用insert()函数插入元素到unordered_set中。
- 删除元素:使用erase()函数删除指定元素或范围内的元素。
- 查找元素:使用find()函数查找指定元素,如果找到则返回指向该元素的迭代器,否则返回end()迭代器。
- 遍历集合:使用迭代器或范围-based for循环遍历unordered_set中的所有元素。
以下是一个简单的示例代码,展示了unordered_set的基本用法:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet;
// 插入元素
mySet.insert(5);
mySet.insert(2);
mySet.insert(8);
// 遍历集合
for (const auto& element : mySet) {
std::cout << element << " ";
}
std::cout << std::endl;
// 查找元素
auto it = mySet.find(2);
if (it != mySet.end()) {
std::cout << "Found element: " << *it << std::endl;
}
// 删除元素
mySet.erase(5);
return 0;
}
```
以上代码将输出:
```
8 2 5
Found element: 2
```
希望能帮到你!如果有任何问题,请随时提问。
阅读全文
相关推荐
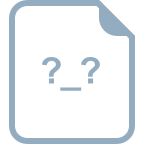
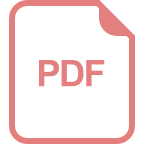
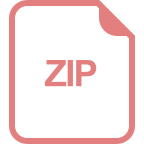













