ds18b20温度传感器stm32 hal库代码
时间: 2023-09-10 12:06:23 浏览: 47
以下是DS18B20温度传感器的STM32 HAL库代码示例:
```c
#include "stm32f4xx_hal.h"
#define DS18B20_PIN GPIO_PIN_0
#define DS18B20_PORT GPIOA
float ds18b20_read_temperature(void);
void ds18b20_reset(void);
void ds18b20_write_bit(uint8_t bit);
uint8_t ds18b20_read_bit(void);
void ds18b20_write_byte(uint8_t byte);
uint8_t ds18b20_read_byte(void);
void ds18b20_reset(void)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(480);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
HAL_Delay(60);
while (!HAL_GPIO_ReadPin(DS18B20_PORT, DS18B20_PIN));
HAL_Delay(420);
}
void ds18b20_write_bit(uint8_t bit)
{
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(bit ? 6 : 60);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
HAL_Delay(bit ? 60 : 6);
}
uint8_t ds18b20_read_bit(void)
{
uint8_t bit = 0;
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_RESET);
HAL_Delay(6);
HAL_GPIO_WritePin(DS18B20_PORT, DS18B20_PIN, GPIO_PIN_SET);
HAL_Delay(9);
bit = HAL_GPIO_ReadPin(DS18B20_PORT, DS18B20_PIN);
HAL_Delay(55);
return bit;
}
void ds18b20_write_byte(uint8_t byte)
{
for (int i = 0; i < 8; i++) {
ds18b20_write_bit(byte & (1 << i));
}
}
uint8_t ds18b20_read_byte(void)
{
uint8_t byte = 0;
for (int i = 0; i < 8; i++) {
byte |= ds18b20_read_bit() << i;
}
return byte;
}
float ds18b20_read_temperature(void)
{
ds18b20_reset();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0x44);
HAL_Delay(750);
ds18b20_reset();
ds18b20_write_byte(0xCC);
ds18b20_write_byte(0xBE);
uint16_t raw_temperature = ds18b20_read_byte() | (ds18b20_read_byte() << 8);
int8_t sign = (raw_temperature & 0x8000) ? -1 : 1;
raw_temperature = (raw_temperature >> 4) & 0x0FFF;
float temperature = (float) raw_temperature / 16.0f * sign;
return temperature;
}
int main(void)
{
HAL_Init();
__HAL_RCC_GPIOA_CLK_ENABLE();
GPIO_InitTypeDef GPIO_InitStruct;
GPIO_InitStruct.Pin = DS18B20_PIN;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_OD;
GPIO_InitStruct.Pull = GPIO_PULLUP;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(DS18B20_PORT, &GPIO_InitStruct);
float temperature = ds18b20_read_temperature();
// Do something with the temperature value
while (1);
}
```
相关推荐
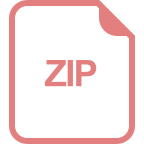
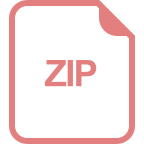
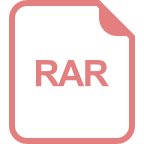














