使用系统调用open read write,完成一个C语言程序:该程序接收两个参数,参数1作为要读写的文件,参数2作为标志位,标识是读还是写。功能完成对参数1所示文件的读写,该文件的内容为 “学号 MYFILE”,其中学号填为自己的学号。
时间: 2023-11-22 15:54:45 浏览: 46
下面是代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
int main(int argc, char *argv[]) {
if (argc != 3) {
printf("Usage: %s <filename> <r/w>\n", argv[0]);
return 1;
}
char *filename = argv[1];
char *mode = argv[2];
int fd;
if (*mode == 'r') {
fd = open(filename, O_RDONLY);
if (fd == -1) {
perror("open");
return 1;
}
char buf[1024];
int len = read(fd, buf, sizeof(buf));
if (len == -1) {
perror("read");
return 1;
}
buf[len] = '\0';
printf("%s\n", buf);
close(fd);
} else if (*mode == 'w') {
fd = open(filename, O_WRONLY | O_CREAT | O_TRUNC, 0666);
if (fd == -1) {
perror("open");
return 1;
}
char *buf = "学号 12345678";
int len = write(fd, buf, strlen(buf));
if (len == -1) {
perror("write");
return 1;
}
close(fd);
} else {
printf("Invalid mode %s\n", mode);
return 1;
}
return 0;
}
```
该程序使用了系统调用`open`、`read`、`write`等来读写文件。首先检查参数数量是否正确,然后根据第二个参数是`r`还是`w`来打开文件,并进行读或写操作。读操作使用`read`函数读取文件内容,写操作使用`write`函数写入指定的字符串。最后关闭文件,返回0表示程序执行成功。
阅读全文
相关推荐


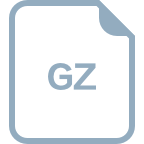









