Python库中还有哪些可以实现TF-IDF、TextRank和LSA三种算法的程序包,并通过实例实现关键词提取。
时间: 2023-06-02 22:03:26 浏览: 222
关于TF-IDF、TextRank和LSA三种算法实现关键词提取的Python程序包,常用的包有:
1. sklearn,它提供了TfidfVectorizer和CountVectorizer等工具,可以实现TF-IDF算法。同时,它也提供了LSA和LDA等主题模型算法。
2. nltk,它是Python自然语言处理的一个常用工具库,其中包含了TextRank算法的实现。
3. gensim,它是一个用于处理文本的Python工具库,提供了LSI(Latent Semantic Indexing,潜在语义分析)和LDA等算法实现。
4. jieba,它是一个中文分词工具库,支持TF-IDF算法。
这些工具库都提供了详细的文档和示例,可以很方便地实现关键词提取。例如,使用sklearn的TfidfVectorizer实现TF-IDF算法的关键词提取代码如下:
```
from sklearn.feature_extraction.text import TfidfVectorizer
texts = ['This is a text', 'Another text', 'Yet another text']
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(texts)
feature_names = vectorizer.get_feature_names()
doc = 0
feature_index = tfidf_matrix[doc,:].nonzero()[1]
tfidf_scores = zip(feature_index, [tfidf_matrix[doc,x] for x in feature_index])
top_keywords = sorted(tfidf_scores, key=lambda x: x[1], reverse=True)[:5]
print(top_keywords)
```
这段代码中,首先使用TfidfVectorizer将文本矩阵转换为TF-IDF矩阵,然后通过get_feature_names方法获取特征名列表,使用nonzero方法获取第0个文本的非零元素下标,通过zip将特征下标和对应的TF-IDF分数打包为元组。最后,使用sorted方法将元组按分数从大到小排序,并选择前5个元组,输出作为关键词。
类似地,使用gensim库的LSI算法实现关键词提取的代码如下:
```
from gensim import corpora
from gensim.models import LsiModel
texts = [['This', 'is', 'a', 'text'], ['Another', 'text'], ['Yet', 'another', 'text']]
dictionary = corpora.Dictionary(texts)
corpus = [dictionary.doc2bow(text) for text in texts]
lsi_model = LsiModel(corpus, num_topics=2)
lsi_matrix = lsi_model[corpus]
doc = 0
top_keywords = sorted(lsi_matrix[doc], key=lambda x: -x[1])[:5]
print(top_keywords)
```
这段代码中,首先使用corpora.Dictionary将文本列表转换为词典,再使用doc2bow方法将每个文本转换为词袋向量表示。然后,使用LsiModel训练得到一个2维的LSI模型,对文本矩阵进行转换得到LSI矩阵。最后,使用sorted方法将LSI矩阵中第0个文本的元素按LSI分数从大到小排序,并选择前5个元素,输出作为关键词。
使用这些工具库,可以很方便地实现关键词提取并进行文本分析。
阅读全文
相关推荐
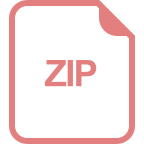
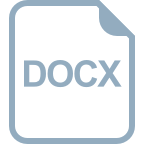

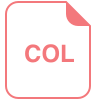
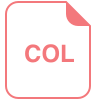
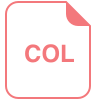
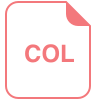
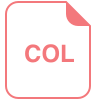
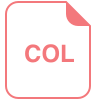
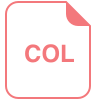
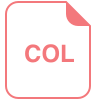
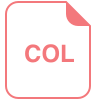
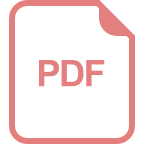
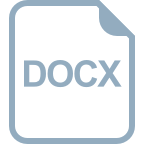
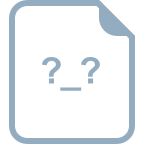
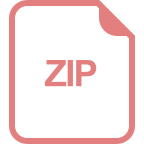
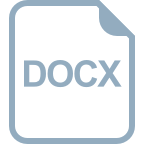