@brief Get a particular bit value from a byte @param b [in] Any byte from which we want a bit value @param p [in] Position of the bit in the byte [0..7] @param n [in] Number of bits we want to get @return The value corresponding the requested bits
时间: 2024-01-20 11:04:08 浏览: 68
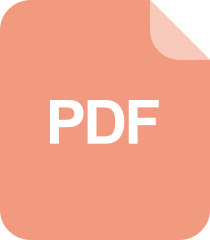
ES9281A-80A-PRO-Product-Brief-v0.2.5.pdf
Here is a possible implementation of the function:
```
unsigned char get_bits(unsigned char b, unsigned char p, unsigned char n) {
unsigned char mask = (1 << n) - 1; // create a mask with n bits set to 1
mask <<= p; // shift the mask to the right position
return (b & mask) >> p; // apply the mask and shift the result to the least significant bits
}
```
This function takes a byte `b`, a position `p` and a number of bits `n` as input, and returns the value of the `n` bits starting at position `p` in the byte. The function first creates a mask with `n` bits set to 1, by left-shifting the value `1` by `n` bits and subtracting 1. The mask is then shifted to the right position by left-shifting it by `p` bits. Finally, the function applies the mask to the byte `b` using the bitwise AND operator (`&`), and shifts the result to the least significant bits by right-shifting it by `p` bits. The resulting value is returned.
阅读全文
相关推荐
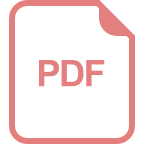
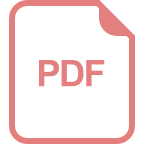















