torch.LongTensor(label).view(-1,1)
时间: 2024-05-25 16:17:49 浏览: 123
This line of code creates a PyTorch LongTensor from the input "label" and then reshapes it into a 2D tensor with one column and as many rows as necessary (-1 means that PyTorch should infer the size of this dimension based on the size of the original tensor and the other specified dimensions).
The reshaping into a 2D tensor with one column is often done to prepare the labels for use in loss functions that expect a 2D tensor of shape (batch_size, num_classes), where each row corresponds to a sample and each column corresponds to a class, with a 1 indicating the correct class for that sample and 0s elsewhere.
相关问题
class ArcFaceLoss(nn.Module): def __init__(self, in_features, out_features, s=35.0, m=0.25): super(ArcFaceLoss, self).__init__() self.s = s self.m = m self.in_features = in_features self.out_features = out_features self.weight = nn.Parameter(torch.FloatTensor(out_features, in_features)) nn.init.xavier_uniform_(self.weight) def forward(self, x, label): cosine = F.linear(F.normalize(x), F.normalize(self.weight)) sine = torch.sqrt(1.0 - torch.pow(cosine, 2)) phi = cosine * torch.cos(torch.tensor(self.m)) - sine * torch.sin(torch.tensor(self.m)) one_hot = torch.zeros(cosine.size(), device=x.device) one_hot.scatter_(1, label.view(-1, 1).long(), 1) logits = (one_hot * phi) + ((1.0 - one_hot) * cosine) logits *= self.s return logits.mean(dim=0).sum()根据这个类写出数学公式
这个类实现了ArcFace损失函数,其数学公式为:
$$L_{arcface}=-\frac{1}{N}\sum_{i=1}^{N}\log\frac{e^{s\cdot\cos(\theta_{y_i}+m)}}{e^{s\cdot\cos(\theta_{y_i}+m)}+\sum_{j\neq y_i}e^{s\cdot\cos\theta_j}}$$
其中,$N$ 是样本数量,$s$ 是一个缩放因子,$m$ 是一个角度差。$\cos\theta_{y_i}$ 是输入特征 $x_i$ 和类别 $y_i$ 对应的权重向量 $w_{y_i}$ 的余弦相似度,$\cos\theta_j$ 是输入特征 $x_i$ 和除了类别 $y_i$ 以外的其他权重向量 $w_j$ 的余弦相似度。$m$ 的作用是增加类间距离,$s$ 的作用是缩放余弦相似度,使得类间距离更加明显。最终损失函数的值为所有样本的损失的平均值。
帮我看看这段代码报错原因: Traceback (most recent call last): File "/home/bder73002/hpy/ConvNextV2_Demo/train+.py", line 274, in <module> train_loss, train_acc = train(model_ft, DEVICE, train_loader, optimizer, epoch,model_ema) File "/home/bder73002/hpy/ConvNextV2_Demo/train+.py", line 48, in train loss = torch.nan_to_num(criterion_train(output, targets)) # 计算loss File "/home/bder73002/anaconda3/envs/python3.9.2/lib/python3.9/site-packages/torch/nn/modules/module.py", line 889, in _call_impl result = self.forward(*input, **kwargs) File "/home/bder73002/hpy/ConvNextV2_Demo/models/losses.py", line 38, in forward index.scatter_(1, target.data.view(-1, 1).type(torch.LongTensor), 1) RuntimeError: Expected index [128, 1] to be smaller than self [16, 8] apart from dimension 1 部分代码如下:cls_num_list = np.zeros(classes) for , label in train_loader.dataset: cls_num_list[label] += 1 criterion_train = LDAMLoss(cls_num_list=cls_num_list, max_m=0.5, s=30) class LDAMLoss(nn.Module): def __init__(self, cls_num_list, max_m=0.5, weight=None, s=30): super(LDAMLoss, self).__init__() m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list)) m_list = m_list * (max_m / np.max(m_list)) m_list = torch.cuda.FloatTensor(m_list) self.m_list = m_list assert s > 0 self.s = s self.weight = weight def forward(self, x, target): index = torch.zeros_like(x, dtype=torch.uint8) # index.scatter_(1, target.data.view(-1, 1), 1) index.scatter_(1, target.data.view(-1, 1).type(torch.LongTensor), 1) index_float = index.type(torch.cuda.FloatTensor) batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(0,1)) batch_m = batch_m.view((-1, 1)) x_m = x - batch_m output = torch.where(index, x_m, x) return F.cross_entropy(self.s*output, target, weight=self.weight)
这个错误是由于 `index` 张量的大小与 `output` 张量的大小不匹配导致的。在你的代码中,`index` 张量的大小是 `[batch_size, classes]`,即每个样本的预测标签的 one-hot 编码,而 `output` 张量的大小是 `[batch_size, features]`,即每个样本的特征向量的大小。因此,如果在 `index.scatter_()` 操作中使用了一个大小为 `[batch_size, 1]` 的张量,则会导致上述错误。
要解决这个问题,你可以将 `index` 的大小更改为 `[batch_size, num_classes]`,其中 `num_classes` 是分类数量。你可以在 `LDAMLoss` 的 `__init__` 方法中将 `num_classes` 作为参数并存储在实例变量中,然后在 `forward` 方法中使用它来创建 `index` 张量。例如:
```
class LDAMLoss(nn.Module):
def __init__(self, cls_num_list, max_m=0.5, weight=None, s=30, num_classes=10):
super(LDAMLoss, self).__init__()
self.num_classes = num_classes
m_list = 1.0 / np.sqrt(np.sqrt(cls_num_list))
m_list = m_list * (max_m / np.max(m_list))
m_list = torch.cuda.FloatTensor(m_list)
self.m_list = m_list
assert s > 0
self.s = s
self.weight = weight
def forward(self, x, target):
index = torch.zeros_like(x, dtype=torch.uint8)
index.scatter_(1, target.data.view(-1, 1).type(torch.LongTensor), 1)
index = index[:, :self.num_classes] # 取前 num_classes 列
index_float = index.type(torch.cuda.FloatTensor)
batch_m = torch.matmul(self.m_list[None, :], index_float.transpose(0,1))
batch_m = batch_m.view((-1, 1))
x_m = x - batch_m
output = torch.where(index, x_m, x)
return F.cross_entropy(self.s*output, target, weight=self.weight)
```
然后在使用 `LDAMLoss` 时,你需要将 `num_classes` 参数传递给它。例如:
```
num_classes = len(train_loader.dataset.classes)
criterion_train = LDAMLoss(cls_num_list=cls_num_list, max_m=0.5, s=30, num_classes=num_classes)
```
阅读全文
相关推荐
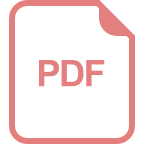
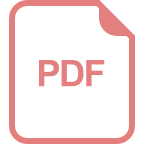
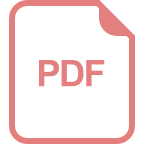












