c语言输出非二叉树中从根到叶子的路径
时间: 2024-12-24 17:38:54 浏览: 2
在C语言中,输出非二叉树(例如图或多叉树)从根节点到叶子节点的路径通常涉及到深度优先搜索(Depth First Search, DFS)算法。对于每个节点,我们遍历它的子节点并记录路径。当遇到叶子节点(即度为0的节点),我们将当前路径打印出来,并回溯到上一个节点继续搜索。
以下是使用递归的一个简单示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* children;
int num_children;
} Node;
// 打印路径函数
void printPath(Node* node, char path[], int depth) {
if (node->num_children == 0) { // 到达叶子节点
for (int i = 0; i < depth; i++) {
printf("%d ", path[i]);
}
printf("\n");
} else {
for (int i = 0; i < node->num_children; i++) {
path[depth] = node->children[i]->data; // 更新路径
printPath(node->children[i], path, depth + 1); // 递归处理子节点
}
}
}
// 深度优先搜索遍历非二叉树
void dfsTraversal(Node* root, char path[]) {
if (root != NULL) {
path[0] = root->data; // 根节点的数据作为路径起始点
printPath(root, path, 1);
}
}
// 创建示例树结构
Node* createTree() {
// 实现创建树的代码...
return NULL;
}
int main() {
Node* tree = createTree();
char path[100]; // 足够大的数组存储路径
dfsTraversal(tree, path);
return 0;
}
```
在这个示例中,你需要自定义`createTree()`函数来构建你的非二叉树结构,然后调用`dfsTraversal()`函数来找出从根到所有叶子节点的路径。
阅读全文
相关推荐
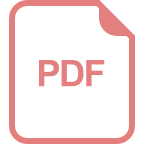
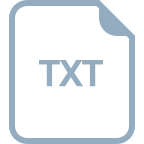
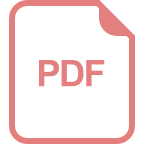















