用c语言写一段“已知三点坐标求其外心坐标”
时间: 2024-02-29 14:51:34 浏览: 22
以下是用C语言实现已知三点坐标求其外心坐标的代码:
```c
#include <stdio.h>
#include <math.h>
struct Point {
double x, y;
};
/* 计算两点之间的距离 */
double distance(struct Point p1, struct Point p2) {
return sqrt(pow(p1.x - p2.x, 2) + pow(p1.y - p2.y, 2));
}
/* 计算三角形面积 */
double triangle_area(struct Point p1, struct Point p2, struct Point p3) {
double a = distance(p1, p2);
double b = distance(p2, p3);
double c = distance(p3, p1);
double p = (a + b + c) / 2.0;
return sqrt(p * (p - a) * (p - b) * (p - c));
}
/* 计算外心坐标 */
struct Point circumcenter(struct Point p1, struct Point p2, struct Point p3) {
double a = p2.x - p1.x, b = p2.y - p1.y, c = p3.x - p1.x, d = p3.y - p1.y;
double e = a * (p1.x + p2.x) + b * (p1.y + p2.y);
double f = c * (p1.x + p3.x) + d * (p1.y + p3.y);
double g = 2.0 * (a * (p3.y - p2.y) - b * (p3.x - p2.x));
if (fabs(g) < 1e-6) { // 三点共线,不存在外心
return (struct Point){-1, -1};
}
double x = (d * e - b * f) / g;
double y = (a * f - c * e) / g;
return (struct Point){x, y};
}
int main() {
struct Point p1 = {0, 0};
struct Point p2 = {3, 0};
struct Point p3 = {0, 4};
double area = triangle_area(p1, p2, p3);
if (area < 1e-6) { // 三点共线,不存在外心
printf("三点共线,不存在外心\n");
} else {
struct Point center = circumcenter(p1, p2, p3);
printf("外心坐标为:(%lf, %lf)\n", center.x, center.y);
}
return 0;
}
```
这段代码实现了已知三个点的坐标求其外心坐标的功能。其中,distance函数用于计算两点之间的距离;triangle_area函数用于计算三角形面积;circumcenter函数用于计算外心坐标。在主函数中,我们定义了三个点的坐标,然后先计算三角形面积,如果面积小于一个极小值,则判定三点共线,不存在外心;否则,调用circumcenter函数计算外心坐标,并输出结果。
相关推荐
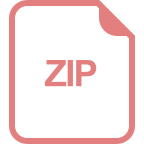














