#include stdlib.h main() char *p: *p=(char*)malloc(100); gets(p);
时间: 2023-11-19 07:05:07 浏览: 29
这段代码有一些问题。
首先,在 `main()` 函数中,应该声明返回值类型为 `int`。因此应该写成 `int main()`。
其次,在第二行中,指针变量 `p` 的类型应该写在变量名前面,即应该写成 `char *p`。
然后,在第三行中,应该为 `p` 分配内存空间,而不是将 `p` 的值赋为 `malloc(100)`。正确的写法是 `p = (char*)malloc(100)`。
最后,在第四行中,使用 `gets()` 函数存在安全问题,因为它无法限制输入的长度,可能会导致缓冲区溢出攻击。应该使用更加安全的函数,比如 `fgets()`。
因此,正确的代码应该是这样的:
```
#include <stdio.h>
#include <stdlib.h>
int main() {
char *p;
p = (char*)malloc(100);
fgets(p, 100, stdin);
printf("You entered: %s\n", p);
free(p);
return 0;
}
```
相关问题
#include<stdio.h> #include<ctype.h> #include<stdlib.h> #include<string.h> char *str(char *name){ char *s1="hello "; strcat(s1,name); return s1; } int main(){ char*name; name=(char*)malloc(20); printf("输入你的名字:"); gets(name); printf("%s",str(name)); }如何修改代码
在您提供的代码中,存在几个需要进行修改的地方。首先,`gets()` 函数是不安全的,已被废弃,应该使用更安全的替代函数 `fgets()`。其次,在 `str()` 函数中,您将字符串常量 `s1` 的地址返回,这是不安全的,因为 `s1` 是一个局部变量,函数结束后会被销毁。下面是修改后的代码:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
char* str(const char* name) {
char* s1 = malloc(strlen(name) + 7); // 为新字符串分配足够的内存
strcpy(s1, "hello ");
strcat(s1, name);
return s1;
}
int main() {
char name[20];
printf("输入你的名字: ");
fgets(name, sizeof(name), stdin); // 使用fgets()获取用户输入
name[strcspn(name, "\n")] = '\0'; // 去除输入字符串中的换行符
printf("%s", str(name));
free(str(name)); // 释放通过malloc分配的内存
return 0;
}
```
这样修改后的代码更安全,避免了不安全的函数和内存问题。同时,我们也对用户输入的字符串进行了处理,去除了可能存在的换行符。请注意在使用完成后释放通过 `malloc` 分配的内存,以避免内存泄漏。
#include <stdio.h> #include <stdlib.h> static char buff[256]; static char* string; int main() { printf("Please input your string:"); gets(string); printf("\nYour string is:%s\n",string); }
这段代码是一个简单的C程序,它的功能是从标准输入中读取一行字符串并输出。
然而,这段代码存在一个严重的问题:它使用了不安全的gets函数来读取输入,可能会导致缓冲区溢出漏洞。正确的做法是使用fgets函数来代替gets函数,限制读取的字符数量。
另外,这段代码声明了一个静态的字符数组buff,但是却没有使用它。而指针变量string却没有被初始化,使用时会导致未定义的行为。应该将string指向buff数组,或者使用动态内存分配函数malloc来为string分配内存。
改进后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_LEN 256
int main() {
char buff[MAX_LEN];
char *string = (char*)malloc(MAX_LEN * sizeof(char));
printf("Please input your string:");
fgets(buff, MAX_LEN, stdin);
sscanf(buff, "%s", string); // 去掉字符串末尾的换行符
printf("\nYour string is:%s\n", string);
free(string);
return 0;
}
```
相关推荐
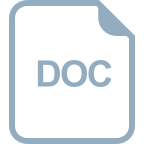
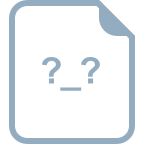
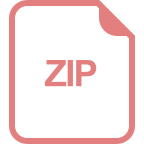












