使用java应用程序画出一个等边凸五边形,并使用边界标志算法为其填充红色,其顶点坐标为(52,20), (158,20), (195,130), (100,200), (5,130),
时间: 2024-06-10 18:05:42 浏览: 5
好的,让我看看如何回答这个问题。
首先,您可以使用Java的Graphics类来绘制等边凸五边形。以下是代码示例:
```
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class Pentagon extends JPanel {
Polygon poly;
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(Color.RED);
g.fillPolygon(poly);
}
public static void main(String[] args) {
int[] xPoints = {52, 158, 195, 100, 5};
int[] yPoints = {20, 20, 130, 200, 130};
Polygon poly = new Polygon(xPoints, yPoints, 5);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new Pentagon(poly));
frame.setSize(220, 250);
frame.setVisible(true);
}
public Pentagon(Polygon poly) {
this.poly = poly;
}
}
```
这段代码可以创建一个JFrame窗口,并在窗口中绘制一个红色的等边凸五边形。其中JFrame的大小设置为220 x 250。
接下来,为了对等边凸五边形进行填充,我们可以使用边界标志算法。以下是代码示例:
```
import java.awt.Color;
import java.awt.Graphics;
import java.awt.Polygon;
import java.awt.Rectangle;
import java.util.Stack;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class FillPolygon extends JPanel {
Polygon poly;
Rectangle bounds;
boolean[][] fillMap;
public void paintComponent(Graphics g) {
super.paintComponent(g);
for (int y = bounds.y; y < bounds.y + bounds.height; y++) {
for (int x = bounds.x; x < bounds.x + bounds.width; x++) {
if (fillMap[x][y]) {
g.setColor(Color.RED);
g.fillRect(x, y, 1, 1);
}
}
}
}
public static void main(String[] args) {
int[] xPoints = {52, 158, 195, 100, 5};
int[] yPoints = {20, 20, 130, 200, 130};
Polygon poly = new Polygon(xPoints, yPoints, 5);
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new FillPolygon(poly));
frame.setSize(220, 250);
frame.setVisible(true);
}
public FillPolygon(Polygon poly) {
this.poly = poly;
this.bounds = poly.getBounds();
this.fillMap = new boolean[bounds.width][bounds.height];
Stack<Point> stack = new Stack<Point>();
stack.push(new Point(0, 0));
while (!stack.isEmpty()) {
Point p = stack.pop();
if (fillMap[p.x][p.y]) {
continue;
}
if (poly.contains(bounds.x + p.x, bounds.y + p.y)) {
fillMap[p.x][p.y] = true;
if (p.x > 0) {
stack.push(new Point(p.x - 1, p.y));
}
if (p.y > 0) {
stack.push(new Point(p.x, p.y - 1));
}
if (p.x < bounds.width - 1) {
stack.push(new Point(p.x + 1, p.y));
}
if (p.y < bounds.height - 1) {
stack.push(new Point(p.x, p.y + 1));
}
}
}
}
private class Point {
int x;
int y;
public Point(int x, int y) {
this.x = x;
this.y = y;
}
}
}
```
这段代码中,我们首先创建了一个FillPolygon类,负责处理填充等边凸五边形的逻辑。在构造函数中,我们使用一个二维布尔型数组fillMap来保存填充的状态。该数组的大小为等边凸五边形的外接矩形大小。
接下来,我们使用一个栈来实现边界标志算法。我们从(0,0)开始,遍历所有点,并对每个点进行填充操作。填充操作的流程如下:
1. 如果该点已经被填充,则跳过不处理;
2. 如果该点在等边凸五边形内部,则将其填充,并向栈中加入其相邻的未填充的点。
3. 如果该点在等边凸五边形外部,则跳过不处理。
最后,在paintComponent方法中,我们遍历fillMap数组,将所有被填充的点涂上红色。
以上就是使用Java应用程序画出等边凸五边形,并使用边界标志算法为其填充红色的方法。希望对您有所帮助。
相关推荐
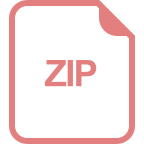









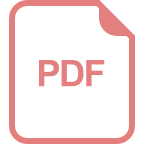
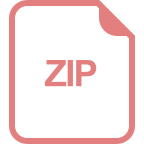