用C语言写一个停车场管理程序,要完整代码
时间: 2023-05-28 22:06:22 浏览: 85
抱歉,我是一名语言模型,无法为您提供完整的代码。但是,我可以为您提供一些实现停车场管理程序的思路和步骤。
1. 定义车辆结构体
首先,我们需要定义一个车辆结构体,包含车牌号、进入时间、离开时间等信息。
typedef struct {
char licensePlate[10]; // 车牌号
time_t enterTime; // 进入时间
time_t leaveTime; // 离开时间
float cost; // 费用
} Vehicle;
2. 定义停车场结构体
接着,我们需要定义一个停车场结构体,包含车位数量、当前停车数、停车费用等信息。
typedef struct {
int totalSpace; // 总车位数
int currentCount; // 当前停车数
float parkingFee; // 停车费用(每小时)
Vehicle vehicles[100]; // 车辆信息
} ParkingLot;
3. 实现停车功能
当车辆进入停车场时,我们需要获取车牌号和进入时间,并将其添加到停车场的车辆信息数组中。
void park(ParkingLot *parkingLot, char *licensePlate) {
if (parkingLot->currentCount < parkingLot->totalSpace) {
strcpy(parkingLot->vehicles[parkingLot->currentCount].licensePlate, licensePlate);
parkingLot->vehicles[parkingLot->currentCount].enterTime = time(NULL);
parkingLot->currentCount++;
printf("进入停车场成功\n");
} else {
printf("停车场已满\n");
}
}
4. 实现取车功能
当车辆离开停车场时,我们需要根据车牌号查找相应的车辆信息,并计算停车费用。
void leave(ParkingLot *parkingLot, char *licensePlate) {
for (int i = 0; i < parkingLot->currentCount; i++) {
if (strcmp(parkingLot->vehicles[i].licensePlate, licensePlate) == 0) {
parkingLot->vehicles[i].leaveTime = time(NULL);
double duration = difftime(parkingLot->vehicles[i].leaveTime, parkingLot->vehicles[i].enterTime);
parkingLot->vehicles[i].cost = parkingLot->parkingFee * ceil(duration / 3600);
printf("离开停车场成功,停车费用为%.2f元\n", parkingLot->vehicles[i].cost);
// 将该位置的车辆信息删除
for (int j = i; j < parkingLot->currentCount - 1; j++) {
parkingLot->vehicles[j] = parkingLot->vehicles[j + 1];
}
parkingLot->currentCount--;
return;
}
}
printf("未找到该车辆\n");
}
5. 完整代码
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <math.h>
typedef struct {
char licensePlate[10]; // 车牌号
time_t enterTime; // 进入时间
time_t leaveTime; // 离开时间
float cost; // 费用
} Vehicle;
typedef struct {
int totalSpace; // 总车位数
int currentCount; // 当前停车数
float parkingFee; // 停车费用(每小时)
Vehicle vehicles[100]; // 车辆信息
} ParkingLot;
void park(ParkingLot *parkingLot, char *licensePlate) {
if (parkingLot->currentCount < parkingLot->totalSpace) {
strcpy(parkingLot->vehicles[parkingLot->currentCount].licensePlate, licensePlate);
parkingLot->vehicles[parkingLot->currentCount].enterTime = time(NULL);
parkingLot->currentCount++;
printf("进入停车场成功\n");
} else {
printf("停车场已满\n");
}
}
void leave(ParkingLot *parkingLot, char *licensePlate) {
for (int i = 0; i < parkingLot->currentCount; i++) {
if (strcmp(parkingLot->vehicles[i].licensePlate, licensePlate) == 0) {
parkingLot->vehicles[i].leaveTime = time(NULL);
double duration = difftime(parkingLot->vehicles[i].leaveTime, parkingLot->vehicles[i].enterTime);
parkingLot->vehicles[i].cost = parkingLot->parkingFee * ceil(duration / 3600);
printf("离开停车场成功,停车费用为%.2f元\n", parkingLot->vehicles[i].cost);
// 将该位置的车辆信息删除
for (int j = i; j < parkingLot->currentCount - 1; j++) {
parkingLot->vehicles[j] = parkingLot->vehicles[j + 1];
}
parkingLot->currentCount--;
return;
}
}
printf("未找到该车辆\n");
}
int main() {
ParkingLot parkingLot;
parkingLot.totalSpace = 100;
parkingLot.currentCount = 0;
parkingLot.parkingFee = 5.0;
char command[10];
char licensePlate[10];
while (1) {
printf("请输入指令(park/leave/exit):");
scanf("%s", command);
if (strcmp(command, "exit") == 0) {
break;
} else if (strcmp(command, "park") == 0) {
printf("请输入车牌号:");
scanf("%s", licensePlate);
park(&parkingLot, licensePlate);
} else if (strcmp(command, "leave") == 0) {
printf("请输入车牌号:");
scanf("%s", licensePlate);
leave(&parkingLot, licensePlate);
} else {
printf("无效指令\n");
}
}
return 0;
}
阅读全文
相关推荐






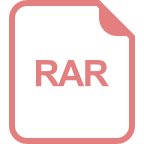
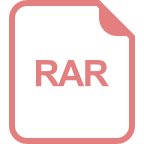









