C语言:中缀表达式求值,只包括加法和乘法
时间: 2024-02-05 20:03:03 浏览: 165
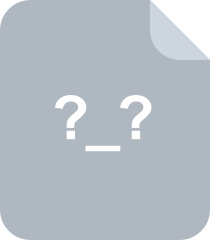
关于中缀表达式求值c语言
中缀表达式求值可以使用栈来实现。具体方法如下:
1. 定义两个栈,一个存放运算符,一个存放操作数。
2. 从左到右遍历中缀表达式,遇到操作数直接入操作数栈。
3. 遇到运算符时,先将其与运算符栈的栈顶元素比较优先级。
4. 如果当前运算符优先级大于栈顶运算符优先级,则直接入栈。
5. 如果当前运算符优先级小于或等于栈顶运算符优先级,则弹出栈顶运算符和两个操作数进行计算,并将结果入操作数栈,直到当前运算符可以入栈。
6. 遍历完表达式后,如果运算符栈不为空,则依次弹出运算符和两个操作数进行计算,直到运算符栈为空。
下面是一个示例代码,只包括加法和乘法:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int top;
int data[100];
} Stack;
void push(Stack *s, int x) {
s->data[++s->top] = x;
}
int pop(Stack *s) {
return s->data[s->top--];
}
int peek(Stack *s) {
return s->data[s->top];
}
int is_empty(Stack *s) {
return s->top == -1;
}
int is_operator(char c) {
return c == '+' || c == '*';
}
int evaluate(char *expr) {
Stack op_stack, num_stack;
op_stack.top = num_stack.top = -1;
for (int i = 0; expr[i] != '\0'; i++) {
char c = expr[i];
if (is_operator(c)) {
while (!is_empty(&op_stack) && peek(&op_stack) != '(' && (c == '*' || peek(&op_stack) == '+')) {
int b = pop(&num_stack);
int a = pop(&num_stack);
char op = pop(&op_stack);
int res = (op == '+') ? a + b : a * b;
push(&num_stack, res);
}
push(&op_stack, c);
} else if (c == '(') {
push(&op_stack, c);
} else if (c == ')') {
while (!is_empty(&op_stack) && peek(&op_stack) != '(') {
int b = pop(&num_stack);
int a = pop(&num_stack);
char op = pop(&op_stack);
int res = (op == '+') ? a + b : a * b;
push(&num_stack, res);
}
pop(&op_stack);
} else {
int num = c - '0';
push(&num_stack, num);
}
}
while (!is_empty(&op_stack)) {
int b = pop(&num_stack);
int a = pop(&num_stack);
char op = pop(&op_stack);
int res = (op == '+') ? a + b : a * b;
push(&num_stack, res);
}
return pop(&num_stack);
}
int main() {
char expr[100];
printf("Enter an infix expression (only + and * operators): ");
scanf("%s", expr);
int res = evaluate(expr);
printf("Result: %d\n", res);
return 0;
}
```
输入示例:(1+2)*3+4*5
输出示例:Result: 23
阅读全文
相关推荐


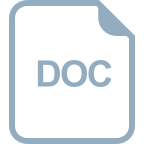
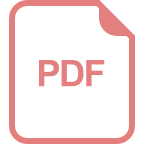
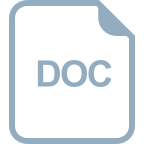


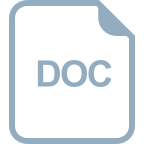
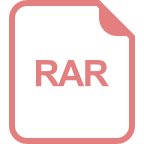
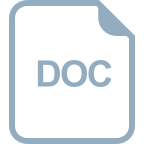
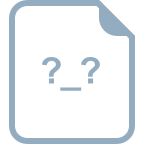
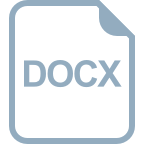
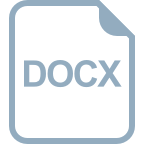
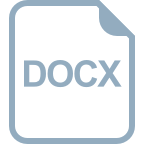
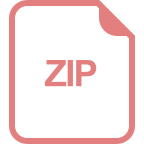
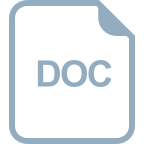