stm32 hal库 bootloader程序代码
时间: 2023-09-02 14:09:02 浏览: 179
以下是一个简单的基于HAL库的STM32 bootloader程序代码,可以用于在芯片上下载和更新应用程序。
```c
#include "main.h"
#include "stm32f1xx_hal.h"
#define FLASH_START_ADDRESS 0x08000000
#define FLASH_END_ADDRESS 0x08010000
typedef void (*pFunction)(void);
void bootloader(void);
void jump_to_application(uint32_t address);
int main(void)
{
HAL_Init();
__HAL_RCC_GPIOC_CLK_ENABLE();
GPIO_InitTypeDef GPIO_InitStruct = {0};
GPIO_InitStruct.Pin = GPIO_PIN_13;
GPIO_InitStruct.Mode = GPIO_MODE_INPUT;
GPIO_InitStruct.Pull = GPIO_NOPULL;
HAL_GPIO_Init(GPIOC, &GPIO_InitStruct);
if (HAL_GPIO_ReadPin(GPIOC, GPIO_PIN_13) == GPIO_PIN_RESET)
{
bootloader(); // Enter bootloader mode
}
else
{
jump_to_application(FLASH_START_ADDRESS); // Jump to application
}
}
void bootloader(void)
{
// Perform any necessary initialization
// Wait for data to arrive over the communication interface (e.g. UART)
while (/* no data received */);
// Erase the flash memory sectors where the application will be written
FLASH_EraseInitTypeDef eraseInitStruct = {0};
eraseInitStruct.TypeErase = FLASH_TYPEERASE_SECTORS;
eraseInitStruct.Sector = FLASH_SECTOR_0;
eraseInitStruct.NbSectors = FLASH_SECTOR_TOTAL;
eraseInitStruct.VoltageRange = FLASH_VOLTAGE_RANGE_3;
uint32_t error = 0;
HAL_FLASH_Unlock();
if (HAL_FLASHEx_Erase(&eraseInitStruct, &error) != HAL_OK)
{
// Handle error
}
HAL_FLASH_Lock();
// Receive data and write it to the flash memory
uint32_t address = FLASH_START_ADDRESS;
while (/* data received */)
{
HAL_FLASH_Unlock();
if (HAL_FLASH_Program(FLASH_TYPEPROGRAM_WORD, address, /* data */) != HAL_OK)
{
// Handle error
}
HAL_FLASH_Lock();
address += sizeof(uint32_t);
}
// Jump to application
jump_to_application(FLASH_START_ADDRESS);
}
void jump_to_application(uint32_t address)
{
pFunction appEntry = (pFunction) *(__IO uint32_t*) (address + 4);
__set_MSP(*(__IO uint32_t*) address);
appEntry();
}
```
这个程序会在启动时检查GPIOC的第13个引脚,如果它被拉低,则进入bootloader模式,否则直接跳转到应用程序。在bootloader模式下,程序会等待从通信接口(如UART)接收数据,并将其写入flash存储器中。最后,程序会跳转到应用程序的入口点。请注意,这个程序只是一个基础版本,实际情况下可能需要进行更多的错误检查和容错处理。
阅读全文
相关推荐
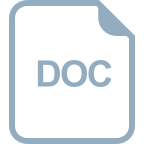
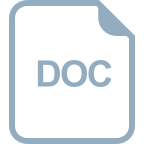
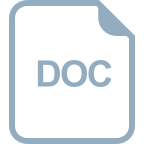






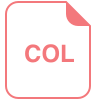



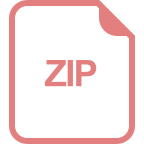
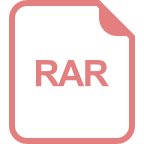
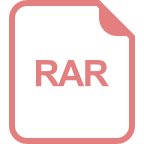
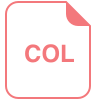

