java 获取freemarker模板路径
时间: 2023-08-10 15:02:23 浏览: 62
要获取Freemarker模板的路径,可以使用以下代码:
```java
Configuration cfg = new Configuration(Configuration.VERSION_2_3_30);
cfg.setClassForTemplateLoading(getClass(), "/templates");
Template template = cfg.getTemplate("myTemplate.ftl");
String templatePath = template.getName();
```
这里的`/templates`是模板文件夹的相对路径,可以根据实际情况修改。`getClass()`方法返回当前类的Class对象,用于指定模板文件夹的位置。`getTemplate("myTemplate.ftl")`方法用于获取模板文件,`getName()`方法返回模板文件的名称和路径。
相关问题
获取freemarker配置的路径
在使用Freemarker时,你需要在项目中指定Freemarker的配置文件,然后在代码中获取配置文件的路径。通常情况下,你可以按照以下步骤来获取Freemarker配置文件的路径:
1. 在项目中创建Freemarker配置文件,例如:`freemarker.properties` 或 `freemarker.xml`。
2. 在代码中获取配置文件的路径,例如:
```java
String path = this.getClass().getClassLoader().getResource("freemarker.properties").getPath();
```
如果你的配置文件是xml格式的,也可以这样获取:
```java
String path = this.getClass().getClassLoader().getResource("freemarker.xml").getFile();
```
注意:上述代码中的`this.getClass().getClassLoader().getResource()`方法会根据类加载器的方式来查找资源,通常情况下会在classpath目录下查找,因此你需要将配置文件放在classpath目录下。
另外,你也可以使用Freemarker提供的`Configuration`类来获取配置文件的路径,例如:
```java
Configuration cfg = new Configuration(Configuration.VERSION_2_3_29);
cfg.setClassForTemplateLoading(this.getClass(), "/"); // 设置Freemarker模板文件的加载路径
String path = cfg.getTemplate("freemarker.properties").getName();
```
其中,`setClassForTemplateLoading()`方法用于指定Freemarker模板文件的加载路径,`getTemplate()`方法用于获取指定的模板文件。
获取springboot中配置的freemarker路径
在Spring Boot中,可以通过在application.properties或application.yml中配置freemarker.template-loader-path属性来指定FreeMarker模板文件的位置。可以使用以下代码从配置文件中获取该属性的值:
```java
@Value("${spring.freemarker.template-loader-path}")
private String templateLoaderPath;
```
然后,您可以在代码中使用templateLoaderPath变量来访问FreeMarker模板文件的位置。
相关推荐
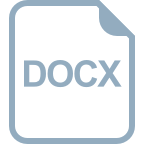
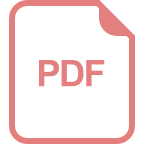
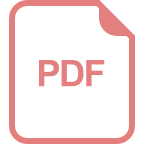












