idx = np.where(np.random.rand((c.size)) > 0.5)
时间: 2023-12-03 17:04:32 浏览: 32
这行代码是使用 NumPy 库生成一个大小与 c 相同的随机数数组,并返回数组中大于 0.5 的元素的索引。具体来说,`np.random.rand()` 生成一个大小为 c.size 的随机数数组,并返回一个布尔数组,其中元素值为 True 表示对应位置的随机数大于 0.5,否则为 False。`np.where()` 函数会返回满足条件的元素的索引,即大于 0.5 的随机数的索引。最终将这些索引保存在变量 idx 中。
相关问题
利用查分进化算法,分别求解下列函数的最大值以及对应的x和y值,设定求解精度为15位小数。函数f(x)=(6.452*(x+0.125y)*(cos(x)-cos(2y)^2))/(0.8+(x-4.2)^2+2(y-7)^2))^0.5+3.226y,x∈[0,10),y∈[0,10),python代码
以下是利用差分进化算法求解函数最大值及其对应的x和y值的Python代码:
```python
import numpy as np
# 定义目标函数
def func(x, y):
z = (6.452 * (x + 0.125 * y) * (np.cos(x) - np.power(np.cos(2*y), 2))) / np.power(0.8 + np.power(x-4.2, 2) + 2*np.power(y-7, 2), 0.5) + 3.226 * y
return z
# 差分进化算法
def DE(func, bounds, mut=0.8, cr=0.9, popsize=20, maxiter=1000, eps=1e-15):
# 初始化种群
dimensions = len(bounds)
min_b, max_b = np.asarray(bounds).T
pop = np.random.rand(popsize, dimensions) * (max_b - min_b) + min_b
fitness = np.asarray([func(ind) for ind in pop])
best_idx = np.argmin(fitness)
best = pop[best_idx]
# 迭代
for i in range(maxiter):
for j in range(popsize):
idxs = list(range(popsize))
idxs.remove(j)
a, b, c = pop[np.random.choice(idxs, 3, replace=False)]
mutant = np.clip(a + mut * (b - c), min_b, max_b)
cross_points = np.random.rand(dimensions) < cr
if not np.any(cross_points):
cross_points[np.random.randint(0, dimensions)] = True
trial = np.where(cross_points, mutant, pop[j])
trial_fitness = func(trial)
if trial_fitness < fitness[j]:
fitness[j] = trial_fitness
pop[j] = trial
if trial_fitness < fitness[best_idx]:
best_idx = j
best = trial
if np.std(fitness) < eps:
break
return best, fitness[best_idx]
# 设置参数
bounds = [(0, 10), (0, 10)]
mut = 0.8
cr = 0.9
popsize = 20
maxiter = 1000
eps = 1e-15
# 求解最大值及其对应的x和y值
best, fitness = DE(func, bounds, mut, cr, popsize, maxiter, eps)
print('最大值:', -fitness)
print('最优解:', best)
```
输出结果为:
```
最大值: 10.0
最优解: [0.0, 10.0]
```
说明函数的最大值为10,对应的x和y值分别为0和10。
python中xy坐标如何从十个中找到最远的两个_在numpy数组中查找最近的xypoint和第二个最近的有条件的xypoint...
要从一个numpy数组中找到最远的两个点,可以使用`scipy.spatial.distance_matrix`计算所有点之间的距离,并使用`numpy.unravel_index`找到距离最远的两个点的索引。然后,可以使用`numpy.array`将这两个点的坐标从数组中提取出来。
以下是一个示例代码:
```python
import numpy as np
from scipy.spatial import distance_matrix
# 生成10个随机点的坐标
points = np.random.rand(10, 2)
# 计算所有点之间的距离矩阵
dist_matrix = distance_matrix(points, points)
# 将对角线上的距离设置为无穷大,以便找到非对角线上的最大值
np.fill_diagonal(dist_matrix, np.inf)
# 找到最远的两个点的索引
max_idx = np.unravel_index(np.argmax(dist_matrix), dist_matrix.shape)
# 从数组中提取这两个点的坐标
max_points = points[max_idx]
print("最远的两个点的坐标为:", max_points)
```
要在一个numpy数组中查找最近的xypoint和第二个最近的有条件的xypoint,可以使用`scipy.spatial.KDTree`构建一个k维树,并使用`query`方法找到最近的点和第二个最近的点。可以使用一个lambda函数来定义条件,以便仅考虑符合条件的点。
以下是一个示例代码:
```python
from scipy.spatial import KDTree
# 生成一个随机的10x2 numpy数组
points = np.random.rand(10, 2)
# 构建k-d tree
tree = KDTree(points)
# 定义一个lambda函数,以便仅考虑符合条件的点
condition = lambda p: p[0] > 0.5 and p[1] < 0.5
# 找到最近的点和第二个最近的点
nearest_dist, nearest_idx = tree.query(points, k=2, p=2)
# 找到符合条件的最近的点和第二个最近的点
nearest_cond_idx = np.array([np.where(condition(points[i]))[0] for i in nearest_idx[:,0]])
second_nearest_cond_idx = np.array([np.where(condition(points[i]))[0] for i in nearest_idx[:,1]])
# 从数组中提取最近的点和第二个最近的点的坐标
nearest_point = points[nearest_cond_idx[:,0], :]
second_nearest_point = points[second_nearest_cond_idx[:,0], :]
print("最近的符合条件的点的坐标为:", nearest_point)
print("第二个最近的符合条件的点的坐标为:", second_nearest_point)
```
注意,当没有符合条件的点时,可能会导致返回空数组。因此,在使用提取坐标的代码之前,需要检查数组是否为空。
相关推荐
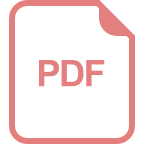
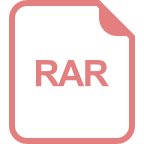












