能写出这个算法的python代码吗
时间: 2023-12-22 17:02:54 浏览: 65
当然可以,以下是一份简单的Python代码实现:
```python
import numpy as np
def DE_with_Strategy_Adaptation(fobj, bounds, npop=50, F=0.5, CR=0.8, ngen=100):
# 初始化种群
pop = np.random.uniform(bounds[0], bounds[1], (npop, len(bounds)))
# 初始化适应度
fitness = np.array([fobj(ind) for ind in pop])
# 初始化最优解和适应度
best_idx = np.argmin(fitness)
best = pop[best_idx]
fbest = fitness[best_idx]
for i in range(ngen):
# 自适应调整F和CR
if i > 0:
F = np.mean(F_history[-3:])
CR = np.mean(CR_history[-3:])
# 选择变异策略
if i > 0:
if np.random.rand() < 0.1:
strategy = np.random.choice(['best', 'rand', 'current'])
else:
strategy = strategy_history[-1]
else:
strategy = 'best'
# 变异
if strategy == 'best':
idxs = np.random.choice(npop, 3, replace=False)
x0, x1, x2 = pop[idxs]
v = best + F * (x1 - x2)
elif strategy == 'rand':
idxs = np.random.choice(npop, 3, replace=False)
x0, x1, x2 = pop[idxs]
v = x0 + F * (x1 - x2)
else:
idx = np.random.randint(npop)
x0 = pop[idx]
idxs = np.random.choice(npop, 2, replace=False)
x1, x2 = pop[idxs]
v = x0 + F * (x1 - x2)
# 交叉
mask = np.random.rand(len(bounds)) < CR
mask[np.random.randint(len(bounds))] = True
u = np.where(mask, v, pop[i])
# 选择
fitness_u = fobj(u)
if fitness_u < fitness[i]:
pop[i] = u
fitness[i] = fitness_u
if fitness_u < fbest:
best = u
fbest = fitness_u
# 记录历史
F_history.append(F)
CR_history.append(CR)
strategy_history.append(strategy)
return best, fbest
```
这份代码实现了DE算法的主要流程,包括种群初始化、变异、交叉、选择、自适应调整等步骤,并且加入了策略自适应机制。其中,`fobj`表示目标函数,`bounds`表示变量的上下界,`npop`表示种群大小,`F`和`CR`表示变异和交叉的参数,`ngen`表示迭代次数。代码中还记录了每次迭代的F、CR和策略,方便观察算法的收敛情况。
阅读全文
相关推荐
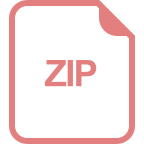
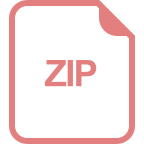
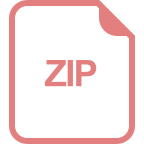














