C++中TArray<uint8>转string怎么做
时间: 2024-05-09 17:17:09 浏览: 12
您好!感谢您提出问题。将TArray<uint8>转换为string可使用以下代码:
```c++
TArray<uint8> ByteArray = {97, 98, 99}; //此为示例TArray<uint8>
FString StringData = FString((const TArray<FUTF8Char>&)ByteArray);
std::string StdStringData(TCHAR_TO_UTF8(*StringData)); //将FString转换为std::string
```
请注意,此方法将TArray<uint8>转换为FString,然后将FString转换为std::string。如果您只需要FString,则可以将上面的第三行忽略。同时,这里假设TArray<uint8>表示ASCII字符序列,如示例中的"abc"。如果TArray<uint8>表示其他编码,则需要进行相应的编码转换。
希望这能够帮到您!
相关问题
C++中string转Tarray<uint8>
在C语言中,string通常是用字符数组表示的,而Tarray<uint8>可以看作是一个无符号整数类型的数组。因此,将string转换为Tarray<uint8>可以通过以下方法实现:
```c
#include <string.h>
#include <stdint.h>
Tarray<uint8> stringToTarray(const char* str) {
// 获取字符串长度
size_t len = strlen(str);
// 创建Tarray<uint8>数组
Tarray<uint8> arr(len);
// 将字符串中的每个字符转换为无符号整数类型并存储到数组中
for (size_t i = 0; i < len; i++) {
arr[i] = (uint8_t) str[i];
}
return arr;
}
```
这段代码首先使用`strlen`函数获取字符串长度,然后创建了一个长度为字符串长度的Tarray<uint8>数组。接着,使用循环将字符串中的每个字符转换为无符号整数类型并存储到数组中,最后返回该数组。
UE4 场景中截取图片并保存(C++实现)
在UE4中,你可以使用C++代码来实现截取场景中的图片并保存到本地。具体步骤如下:
1. 创建一个C++类,并继承自AActor类。命名为"ScreenshotActor"。
2. 在"ScreenshotActor.h"文件中添加以下头文件:
```cpp
#include "Engine/Engine.h"
#include "Kismet/GameplayStatics.h"
#include "Engine/GameViewportClient.h"
#include "Misc/FileHelper.h"
#include "Misc/Paths.h"
```
3. 在"ScreenshotActor.h"文件中添加以下声明:
```cpp
UCLASS()
class YOURPROJECT_API AScreenshotActor : public AActor
{
GENERATED_BODY()
public:
// Sets default values for this actor's properties
AScreenshotActor();
// Take screenshot and save to file
UFUNCTION(BlueprintCallable, Category = "Screenshot")
void TakeScreenshot();
private:
// The resolution multiplier for the screenshot
UPROPERTY(EditAnywhere, Category = "Screenshot")
int32 ResolutionMultiplier = 1;
};
```
4. 在"ScreenshotActor.cpp"文件中添加以下代码:
```cpp
#include "ScreenshotActor.h"
AScreenshotActor::AScreenshotActor()
{
PrimaryActorTick.bCanEverTick = false;
}
void AScreenshotActor::TakeScreenshot()
{
// Get the game viewport client
UGameViewportClient* ViewportClient = GEngine->GameViewport;
if (ViewportClient)
{
// Get the viewport size
FVector2D Size;
ViewportClient->GetViewportSize(Size);
// Calculate the screenshot resolution
int32 ResolutionX = Size.X * ResolutionMultiplier;
int32 ResolutionY = Size.Y * ResolutionMultiplier;
// Take the screenshot
TArray<FColor> Bitmap;
Bitmap.AddUninitialized(ResolutionX * ResolutionY);
ViewportClient->ReadPixels(Bitmap);
// Create the screenshot texture
UTexture2D* Screenshot = UTexture2D::CreateTransient(ResolutionX, ResolutionY);
Screenshot->CompressionSettings = TC_HDR;
Screenshot->SRGB = false;
Screenshot->AddToRoot();
Screenshot->UpdateResource();
// Copy the pixel data to the texture
uint8* MipData = static_cast<uint8*>(Screenshot->PlatformData->Mips[0].BulkData.Lock(LOCK_READ_WRITE));
FMemory::Memcpy(MipData, Bitmap.GetData(), Bitmap.Num() * sizeof(FColor));
Screenshot->PlatformData->Mips[0].BulkData.Unlock();
// Save the screenshot to file
FDateTime Now = FDateTime::Now();
FString Filename = FString::Printf(TEXT("Screenshot_%04d%02d%02d_%02d%02d%02d.png"), Now.GetYear(), Now.GetMonth(), Now.GetDay(), Now.GetHour(), Now.GetMinute(), Now.GetSecond());
FString SaveDirectory = FPaths::GameSavedDir() + TEXT("Screenshots/");
if (!FPaths::DirectoryExists(SaveDirectory))
{
FPlatformFileManager::Get().GetPlatformFile().CreateDirectory(*SaveDirectory);
}
FString FilePath = SaveDirectory + Filename;
FFileHelper::SaveArrayToFile(Screenshot->GetRawImageData(), Screenshot->GetAllocatedSize(), *FilePath);
// Remove the screenshot texture
Screenshot->RemoveFromRoot();
}
}
```
5. 在UE4编辑器中创建一个蓝图,并选择"ScreenshotActor"作为其父类。
6. 在蓝图中添加一个"Take Screenshot"函数,并调用AScreenshotActor::TakeScreenshot()函数。
7. 在场景中放置该蓝图,并运行游戏。当需要截取场景中的图片时,调用蓝图中的"Take Screenshot"函数即可。
注意:在截图之前需要确保场景中的所有元素都已经加载完毕,否则可能会导致截图不完整或者出现其他问题。另外,该实现只适用于PC平台,如果需要支持其他平台,请参考UE4官方文档或者相关论坛。
相关推荐
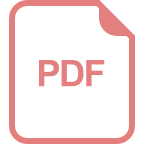
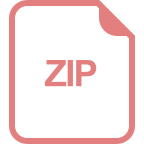
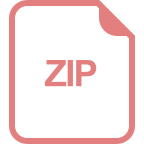









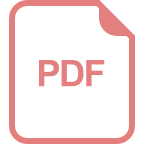
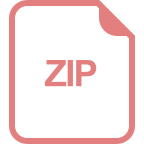
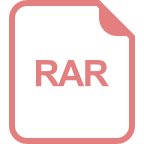
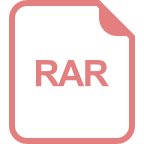