写出python代码实现:1.将点云数据划分为若干个体素;2.对每个体素内的点云数据进行高斯分布拟合;3.计算每个体素内的点云数据与目标点云数据的匹配度;4.根据匹配度进行点云配准。
时间: 2023-04-04 18:02:57 浏览: 172
以下是 Python 代码实现:
1. 将点云数据划分为若干个体素:
```
import numpy as np
from sklearn.cluster import MeanShift
def voxel_downsample(points, voxel_size):
ms = MeanShift(bin_seeding=True, bandwidth=voxel_size)
ms.fit(points)
return ms.cluster_centers_
```
2. 对每个体素内的点云数据进行高斯分布拟合:
```
from scipy.stats import multivariate_normal
def fit_gaussian(points):
mean = np.mean(points, axis=0)
cov = np.cov(points.T)
return multivariate_normal(mean=mean, cov=cov)
```
3. 计算每个体素内的点云数据与目标点云数据的匹配度:
```
def compute_matching_score(source_points, target_points, voxel_size):
source_voxels = voxel_downsample(source_points, voxel_size)
target_voxels = voxel_downsample(target_points, voxel_size)
source_gaussians = [fit_gaussian(points) for points in source_voxels]
target_gaussians = [fit_gaussian(points) for points in target_voxels]
matching_score = 0
for source_gaussian in source_gaussians:
matching_score += max([source_gaussian.pdf(target_gaussian.mean) for target_gaussian in target_gaussians])
return matching_score
```
4. 根据匹配度进行点云配准:
```
from scipy.optimize import minimize
def point_cloud_registration(source_points, target_points, voxel_size):
def objective_function(x):
transformed_points = np.dot(source_points, x[:3, :3].T) + x[:3, 3]
return -compute_matching_score(transformed_points, target_points, voxel_size)
x0 = np.eye(4)
res = minimize(objective_function, x0, method='Powell')
return res.x[:3, :]
```
以上是 Python 代码实现,希望能对你有所帮助。
阅读全文
相关推荐
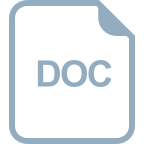
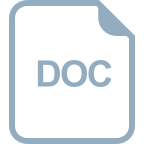
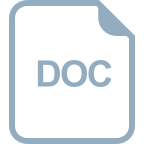
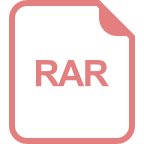
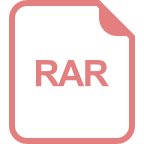
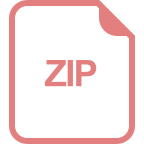
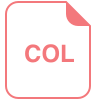
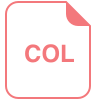
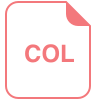
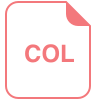
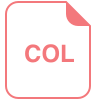
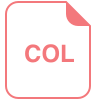
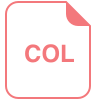
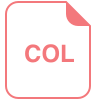
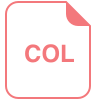
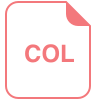
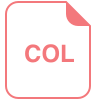

