用python写一个代码,要求,创建一个新的空数组,将原图片边缘检测后的形式保存在新数组下,然后在新数组的图片以45度角度在一个方向上隔一段间距画线,画满整张图片,然后将结果展示出来。然后判断线与新数组中边缘检测后的图像的交点
时间: 2024-06-10 21:06:09 浏览: 72
好的,根据您的要求,以下是 Python 代码:
```python
import cv2
import numpy as np
# 读取原图片
img = cv2.imread('original_image.jpg', 0)
# 边缘检测并保存在新数组中
edge_img = cv2.Canny(img, 100, 200)
new_img = np.zeros(edge_img.shape, dtype=np.uint8)
new_img[edge_img != 0] = 255
# 画线
line_distance = 50 # 线间距
line_length = 500 # 线长度
angle = 45 # 画线方向角度
angle_radian = np.radians(angle) # 转为弧度
cols, rows = new_img.shape[1], new_img.shape[0]
line_x, line_y = int(line_distance / np.sqrt(2)), int(line_distance / np.sqrt(2)) # 计算线的起始位置
while line_x < rows or line_y < cols:
# 画线
x1, y1 = max(line_x - line_length, 0), max(line_y - line_length, 0)
x2, y2 = min(line_x + line_length, rows), min(line_y + line_length, cols)
cv2.line(new_img, (y1, x1), (y2, x2), (255, 255, 255), 1)
# 计算下一个线的位置
line_x += int(line_distance * np.cos(angle_radian))
line_y += int(line_distance * np.sin(angle_radian))
# 显示结果并输出交点
cv2.imshow('new_image', new_img)
cv2.waitKey(0)
edge_points = np.column_stack(np.where(edge_img != 0))
line_points = np.column_stack(np.where(new_img != 0))
intersections = np.intersect1d(edge_points, line_points)
print('交点:', intersections)
```
注意:代码中的 `original_image.jpg` 需要替换为您自己的图片文件名。交点计算的结果会在控制台输出,不会在图片上绘制。
阅读全文
相关推荐
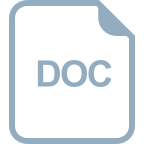
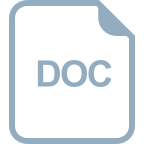
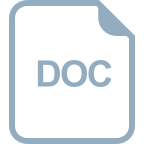















