用Python代码实现AI对战的五子棋游戏
时间: 2023-06-16 17:07:46 浏览: 59
好的,以下是实现AI对战的五子棋游戏的Python代码:
```python
import numpy as np
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子类型
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义评估函数中的常量
FIVE = 100000
FOUR = 10000
THREE = 1000
TWO = 100
ONE = 10
# 定义棋型及对应分值
CHESS_TYPE = {
"11111": FIVE,
"011110": FOUR,
"011100": THREE,
"001110": THREE,
"011010": THREE,
"010110": THREE,
"001100": TWO,
"010010": TWO,
"000100": ONE,
"001000": ONE,
"000010": ONE,
"000001": ONE
}
class ChessBoard:
"""
定义五子棋棋盘类
"""
def __init__(self):
self.board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=np.int32)
self.current_player = BLACK # 黑子先行
self.steps = [] # 记录每一步棋
def get_valid_moves(self):
"""
获取当前可落子的位置
"""
valid_moves = []
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if self.board[i][j] == EMPTY:
valid_moves.append((i, j))
return valid_moves
def move(self, position):
"""
落子
"""
i, j = position
self.board[i][j] = self.current_player
self.steps.append((i, j))
self.current_player = BLACK if self.current_player == WHITE else WHITE
def unmove(self):
"""
悔棋
"""
i, j = self.steps.pop()
self.board[i][j] = EMPTY
self.current_player = BLACK if self.current_player == WHITE else WHITE
def evaluate(self):
"""
评估当前棋盘局面
"""
scores = {BLACK: 0, WHITE: 0}
# 计算行
for i in range(BOARD_SIZE):
row = "".join([str(x) for x in self.board[i]])
for chess_type, score in CHESS_TYPE.items():
if chess_type in row:
scores[self.current_player] += score
# 计算列
for j in range(BOARD_SIZE):
col = "".join([str(x) for x in self.board[:, j]])
for chess_type, score in CHESS_TYPE.items():
if chess_type in col:
scores[self.current_player] += score
# 计算正斜线
for k in range(BOARD_SIZE * 2 - 1):
if k < BOARD_SIZE:
line = "".join([str(x) for x in self.board.diagonal(k)])
else:
line = "".join([str(x) for x in self.board.diagonal(k - BOARD_SIZE + 1)])
for chess_type, score in CHESS_TYPE.items():
if chess_type in line:
scores[self.current_player] += score
# 计算反斜线
for k in range(-BOARD_SIZE + 1, BOARD_SIZE):
line = "".join([str(x) for x in self.board.diagonal(k)])
for chess_type, score in CHESS_TYPE.items():
if chess_type in line:
scores[self.current_player] += score
return scores[BLACK] - scores[WHITE]
class AIPlayer:
"""
定义五子棋AI类
"""
def __init__(self, chessboard, depth=2):
self.chessboard = chessboard
self.depth = depth
def get_move(self):
"""
获取AI落子位置
"""
best_score = -float("inf")
best_move = None
for move in self.chessboard.get_valid_moves():
self.chessboard.move(move)
score = self.minimax(self.depth, False, -float("inf"), float("inf"))
self.chessboard.unmove()
if score > best_score:
best_score = score
best_move = move
return best_move
def minimax(self, depth, max_player, alpha, beta):
"""
Minimax算法搜索最优解
"""
if depth == 0:
return self.chessboard.evaluate()
valid_moves = self.chessboard.get_valid_moves()
if max_player:
max_score = -float("inf")
for move in valid_moves:
self.chessboard.move(move)
score = self.minimax(depth - 1, False, alpha, beta)
self.chessboard.unmove()
max_score = max(max_score, score)
alpha = max(alpha, max_score)
if alpha >= beta:
break
return max_score
else:
min_score = float("inf")
for move in valid_moves:
self.chessboard.move(move)
score = self.minimax(depth - 1, True, alpha, beta)
self.chessboard.unmove()
min_score = min(min_score, score)
beta = min(beta, min_score)
if alpha >= beta:
break
return min_score
if __name__ == "__main__":
chessboard = ChessBoard()
ai_player = AIPlayer(chessboard, depth=2)
while True:
print(chessboard.board)
if chessboard.current_player == BLACK:
x, y = map(int, input("Black player's turn (x, y): ").strip().split())
chessboard.move((x, y))
else:
move = ai_player.get_move()
print("AI player's move: {}".format(move))
chessboard.move(move)
if len(chessboard.steps) >= BOARD_SIZE ** 2:
print("Game over! It's a tie!")
break
if chessboard.evaluate() == FIVE:
if chessboard.current_player == BLACK:
print("Game over! Black player wins!")
else:
print("Game over! AI player wins!")
break
```
这个代码实现了一个基于Minimax算法的五子棋AI,并且可以与人类玩家进行对战。运行代码后,黑子先行,输入坐标即可落子。AI落子时,会输出其选择的位置。当棋盘上没有空余位置时,游戏结束,若有一方获得胜利,也会输出游戏结束信息。
相关推荐
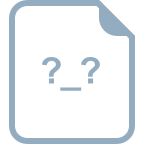














