编写一个带人机对战的五子棋python代码
时间: 2023-10-10 18:16:03 浏览: 104
以下是一个简单的人机对战五子棋的Python代码,使用了minimax算法:
```python
import random
# 创建棋盘
def create_board():
board = []
for i in range(15):
row = []
for j in range(15):
row.append(' ')
board.append(row)
return board
# 打印棋盘
def print_board(board):
print(" ", end='')
for i in range(15):
print(chr(i+ord('a')), end=' ')
print()
for i in range(15):
print("%2d" % (i+1), end=' ')
for j in range(15):
print(board[i][j], end='|')
print()
print(" -----------------------")
# 判断是否胜利
def is_win(board, row, col, player):
# 横向
count = 0
for i in range(15):
if board[row][i] == player:
count += 1
else:
count = 0
if count == 5:
return True
# 纵向
count = 0
for i in range(15):
if board[i][col] == player:
count += 1
else:
count = 0
if count == 5:
return True
# 正对角线
count = 0
for i in range(-4, 5):
if row+i < 0 or row+i >= 15 or col+i < 0 or col+i >= 15:
continue
if board[row+i][col+i] == player:
count += 1
else:
count = 0
if count == 5:
return True
# 反对角线
count = 0
for i in range(-4, 5):
if row+i < 0 or row+i >= 15 or col-i < 0 or col-i >= 15:
continue
if board[row+i][col-i] == player:
count += 1
else:
count = 0
if count == 5:
return True
return False
# 判断是否平局
def is_draw(board):
for i in range(15):
for j in range(15):
if board[i][j] == ' ':
return False
return True
# 估值函数
def evaluate(board):
score = 0
# 横向
for i in range(15):
count = 0
for j in range(15):
if board[i][j] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i][j] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
if count > 1:
score += count*count
# 纵向
for j in range(15):
count = 0
for i in range(15):
if board[i][j] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i][j] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
if count > 1:
score += count*count
# 正对角线
for i in range(15):
count = 0
for j in range(15):
if i+j < 15:
if board[i+j][j] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i+j][j] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
else:
break
if count > 1:
score += count*count
for j in range(1, 15):
count = 0
for i in range(15):
if i+j < 15:
if board[i][i+j] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i][i+j] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
else:
break
if count > 1:
score += count*count
# 反对角线
for i in range(15):
count = 0
for j in range(15):
if i-j >= 0:
if board[i-j][j] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i-j][j] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
else:
break
if count > 1:
score += count*count
for j in range(1, 15):
count = 0
for i in range(15):
if j+i < 15 and i-j >= 0:
if board[i-j][j+i] == ' ':
if count > 1:
score += count*count
count = 0
elif board[i-j][j+i] == 'x':
if count > 0:
score += count*count
count = 0
else:
count += 1
else:
break
if count > 1:
score += count*count
return score
# 递归搜索
def search(board, depth, alpha, beta, player):
if depth == 0:
return None, evaluate(board)
if player == 'x':
best_move = None
best_score = -999999
for i in range(15):
for j in range(15):
if board[i][j] == ' ':
board[i][j] = 'x'
score = search(board, depth-1, alpha, beta, 'o')[1]
board[i][j] = ' '
if score > best_score:
best_score = score
best_move = (i, j)
alpha = max(alpha, best_score)
if beta <= alpha:
return best_move, best_score
return best_move, best_score
else:
best_move = None
best_score = 999999
for i in range(15):
for j in range(15):
if board[i][j] == ' ':
board[i][j] = 'o'
score = search(board, depth-1, alpha, beta, 'x')[1]
board[i][j] = ' '
if score < best_score:
best_score = score
best_move = (i, j)
beta = min(beta, best_score)
if beta <= alpha:
return best_move, best_score
return best_move, best_score
# 人机对战
def play():
board = create_board()
print_board(board)
while True:
# 人下子
row = int(input("请输入行号(1-15): ")) - 1
col = ord(input("请输入列号(a-o): ")) - ord('a')
if board[row][col] != ' ':
print("此处已有棋子,请重新输入!")
continue
board[row][col] = 'o'
print_board(board)
if is_win(board, row, col, 'o'):
print("你赢了!")
break
if is_draw(board):
print("平局!")
break
# AI下子
print("AI正在思考...")
row, col = search(board, 2, -999999, 999999, 'x')[0]
board[row][col] = 'x'
print_board(board)
if is_win(board, row, col, 'x'):
print("AI赢了!")
break
if is_draw(board):
print("平局!")
break
play()
```
以上代码实现了人机对战五子棋,玩家输入行号和列号来下子,AI使用minimax算法来下子。您可以根据自己的需求修改估值函数和搜索深度。
阅读全文
相关推荐
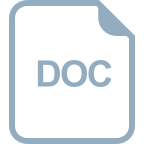
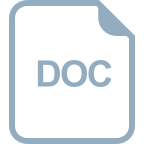
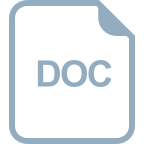





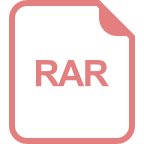
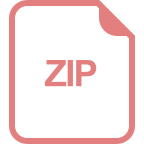
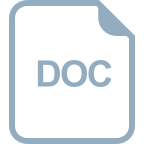







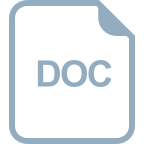