五子棋人机对战系统python
时间: 2023-07-26 20:19:55 浏览: 53
好的,我可以为您提供一些关于五子棋人机对战系统的Python编程思路和建议。
首先,您需要设计一个基本的五子棋游戏界面,可以使用Python的Tkinter模块来创建图形用户界面(GUI)。您可以使用一个棋盘(grid)来表示游戏的场景,并使用不同的颜色来区分不同的棋子。
接下来,您需要编写能够判断游戏胜负的算法。例如,当有五个棋子在同一行、同一列或同一对角线上时,游戏就会结束。这个算法可以使用循环和条件语句来实现。
然后,您需要设计一个能够与玩家交互并下棋的功能。您可以使用鼠标点击事件来实现下棋的功能,并将棋子放置在棋盘的相应位置。同时,您还需要编写一个可以与玩家交替进行下棋的函数。
最后,您需要编写一个能够自动下棋的AI算法。您可以使用基本的博弈树搜索算法或者深度学习算法来实现。具体的实现方法会根据您选择的算法而有所不同。
希望这些建议能够帮助您开始编写五子棋人机对战系统。如果您有任何问题或需要更多的帮助,请随时告诉我。
相关问题
python五子棋人机对战_Python:游戏:五子棋之人机对战
好的,我了解你的问题。你想了解如何使用Python编写五子棋人机对战游戏,对吧?
首先,你需要安装Python的GUI库,如Tkinter或PyQt5,来创建游戏界面。接着,你需要编写五子棋的游戏逻辑。这包括实现棋盘和棋子的绘制、落子、判断胜负等功能。
对于人机对战,你需要实现AI算法来让电脑能够下棋。可以使用经典的博弈树搜索算法,或者深度学习算法来训练神经网络来进行决策。
以下是一个简单的五子棋人机对战游戏的代码示例,供你参考:
```python
import tkinter as tk
import random
class Gobang:
def __init__(self):
self.root = tk.Tk()
self.root.title("五子棋-人机对战")
self.chessboard = Chessboard(self.root, width=500, height=500)
self.chessboard.pack()
self.chessboard.bind("<Button-1>", self.play)
self.player = 1
self.gameover = False
self.ai = AI(self.chessboard)
def play(self, event):
if self.gameover:
return
x, y = self.chessboard.get_index(event.x, event.y)
if self.chessboard.chess_map[x][y] != 0:
return
self.chessboard.draw_chess(x, y, self.player)
self.chessboard.chess_map[x][y] = self.player
if self.check_win(x, y):
self.gameover = True
tk.messagebox.showinfo("游戏结束", "恭喜玩家{}获胜!".format(self.player))
return
self.player = 3 - self.player
self.ai.play()
if self.check_win(self.ai.last_x, self.ai.last_y):
self.gameover = True
tk.messagebox.showinfo("游戏结束", "恭喜电脑获胜!")
def check_win(self, x, y):
directions = [(0, 1), (1, 0), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
tx, ty = x, y
while tx + dx >= 0 and tx + dx < 15 and ty + dy >= 0 and ty + dy < 15 and self.chessboard.chess_map[tx + dx][ty + dy] == self.player:
count += 1
tx += dx
ty += dy
tx, ty = x, y
while tx - dx >= 0 and tx - dx < 15 and ty - dy >= 0 and ty - dy < 15 and self.chessboard.chess_map[tx - dx][ty - dy] == self.player:
count += 1
tx -= dx
ty -= dy
if count >= 5:
return True
return False
def run(self):
self.root.mainloop()
class Chessboard(tk.Canvas):
def __init__(self, master=None, cnf={}, **kw):
super().__init__(master, cnf, **kw)
self.chess_map = [[0 for i in range(15)] for j in range(15)]
self.last_chess = None
self.draw_board()
def draw_board(self):
for i in range(15):
self.create_line(50, 50 + i * 30, 440, 50 + i * 30)
self.create_line(50 + i * 30, 50, 50 + i * 30, 440)
self.create_oval(83, 83, 117, 117, fill="black")
self.create_oval(413, 83, 447, 117, fill="black")
self.create_oval(83, 413, 117, 447, fill="black")
self.create_oval(413, 413, 447, 447, fill="black")
def draw_chess(self, x, y, player):
color = "black" if player == 1 else "white"
self.create_oval(50 + y * 30 - 13, 50 + x * 30 - 13, 50 + y * 30 + 13, 50 + x * 30 + 13, fill=color)
self.last_chess = (x, y)
def get_index(self, x, y):
return (round((y - 50) / 30), round((x - 50) / 30))
class AI:
def __init__(self, chessboard):
self.chessboard = chessboard
self.last_x = -1
self.last_y = -1
def play(self):
empty_list = []
for i in range(15):
for j in range(15):
if self.chessboard.chess_map[i][j] == 0:
empty_list.append((i, j))
if not empty_list:
return
x, y = self.random_play(empty_list)
self.chessboard.draw_chess(x, y, 2)
self.chessboard.chess_map[x][y] = 2
self.last_x, self.last_y = x, y
def random_play(self, empty_list):
return random.choice(empty_list)
if __name__ == "__main__":
game = Gobang()
game.run()
```
这段代码使用了Tkinter库来创建游戏界面,使用了Canvas组件来绘制棋盘和棋子。AI算法使用了随机算法,即在可落子的点中随机选择一个点进行下棋。
当你运行这段代码时,会弹出一个窗口,你可以在窗口中进行五子棋的人机对战游戏。
五子棋人机对战 python
您好!如果您想要开发一个五子棋的人机对战程序,可以使用Python编程语言来实现。下面是一个简单的示例代码,用于展示如何实现基本的五子棋游戏逻辑:
```python
import numpy as np
# 定义棋盘大小为15x15
BOARD_SIZE = 15
# 定义棋盘状态,0表示空,1表示玩家1(黑棋),2表示玩家2(白棋)
board = np.zeros((BOARD_SIZE, BOARD_SIZE), dtype=int)
# 判断输赢的函数
def check_win(player):
# 检查行
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE - 4):
if np.all(board[i, j:j+5] == player):
return True
# 检查列
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE):
if np.all(board[i:i+5, j] == player):
return True
# 检查对角线
for i in range(BOARD_SIZE - 4):
for j in range(BOARD_SIZE - 4):
if np.all(np.diagonal(board[i:i+5, j:j+5]) == player):
return True
# 检查反对角线
for i in range(BOARD_SIZE - 4):
for j in range(4, BOARD_SIZE):
if np.all(np.diagonal(np.fliplr(board[i:i+5, j-4:j+1])) == player):
return True
return False
# 主循环
def main():
current_player = 1
while True:
# 打印当前棋盘状态
print(board)
if current_player == 1:
print("黑棋落子")
else:
print("白棋落子")
# 获取玩家输入的落子位置
x, y = map(int, input("请输入落子位置(以逗号分隔):").split(','))
# 检查输入是否合法
if x < 0 or x >= BOARD_SIZE or y < 0 or y >= BOARD_SIZE or board[x, y] != 0:
print("落子位置无效,请重新输入!")
continue
# 在棋盘上落子
board[x, y] = current_player
# 检查是否获胜
if check_win(current_player):
print("玩家{}获胜!".format(current_player))
break
# 切换玩家
current_player = 3 - current_player
# 启动游戏
main()
```
请注意,上述代码只是一个简单的示例,没有包含任何人机对战的逻辑。如果您想要实现一个具有人机对战功能的五子棋游戏,您需要进一步开发算法来实现AI的决策。
相关推荐
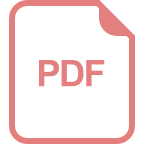
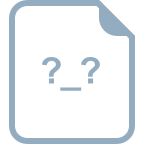
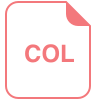









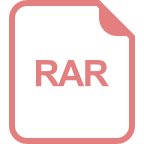
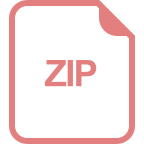