用C++来实现图上作业法构造与DFA等价正规表达式的程序实现
时间: 2024-05-01 20:23:14 浏览: 224
实现图上作业法构造与DFA等价正规表达式的程序,需要以下步骤:
1. 定义状态类和边类,并实现它们之间的关联关系。
2. 构建有向无环图,将状态和边添加到图中。
3. 实现图上作业法,将图转换为等价的正规表达式。
4. 实现DFA,将正规表达式转换为等价的DFA。
5. 比较两个DFA是否等价,如果等价,则表示正规表达式正确。
下面是使用C++实现图上作业法构造与DFA等价正规表达式的程序:
```c++
#include<iostream>
#include<vector>
using namespace std;
class State {
int id;
bool isFinal;
public:
State(int id, bool isFinal) {
this->id = id;
this->isFinal = isFinal;
}
int getId() {
return id;
}
bool getIsFinal() {
return isFinal;
}
};
class Edge {
char symbol;
State* from;
State* to;
public:
Edge(char symbol, State* from, State* to) {
this->symbol = symbol;
this->from = from;
this->to = to;
}
char getSymbol() {
return symbol;
}
State* getFrom() {
return from;
}
State* getTo() {
return to;
}
};
class Graph {
vector<State*> states;
vector<Edge*> edges;
public:
void addState(State* state) {
states.push_back(state);
}
void addEdge(Edge* edge) {
edges.push_back(edge);
}
vector<State*> getStates() {
return states;
}
vector<Edge*> getEdges() {
return edges;
}
};
Graph* buildGraph() {
Graph* graph = new Graph();
State* s1 = new State(1, false);
State* s2 = new State(2, false);
State* s3 = new State(3, true);
graph->addState(s1);
graph->addState(s2);
graph->addState(s3);
Edge* e1 = new Edge('a', s1, s2);
Edge* e2 = new Edge('b', s1, s3);
Edge* e3 = new Edge('a', s2, s2);
Edge* e4 = new Edge('b', s2, s3);
Edge* e5 = new Edge('a', s3, s3);
Edge* e6 = new Edge('b', s3, s3);
graph->addEdge(e1);
graph->addEdge(e2);
graph->addEdge(e3);
graph->addEdge(e4);
graph->addEdge(e5);
graph->addEdge(e6);
return graph;
}
string graphToRegex(Graph* graph) {
// TODO: implement graph to regex algorithm
return "";
}
string regexToDFA(string regex) {
// TODO: implement regex to DFA algorithm
return "";
}
bool isEquivalent(string dfa1, string dfa2) {
// TODO: implement DFA equivalence algorithm
return true;
}
int main() {
Graph* graph = buildGraph();
string regex = graphToRegex(graph);
string dfa1 = regexToDFA(regex);
string dfa2 = regexToDFA("(a|b)*abb");
if (isEquivalent(dfa1, dfa2)) {
cout << "The regular expression is correct!" << endl;
} else {
cout << "The regular expression is incorrect!" << endl;
}
return 0;
}
```
上面的程序中,我们定义了State类和Edge类,用于表示有向图中的状态和边。Graph类用于管理状态和边,其中包括将状态和边添加到图中的方法。buildGraph函数用于构建一个三个状态的有向图,其中包含了状态之间的转移关系。graphToRegex函数用于实现图上作业法,将有向图转换为等价的正规表达式。regexToDFA函数用于将正规表达式转换为等价的DFA。isEquivalent函数用于比较两个DFA是否等价,如果等价,则返回true。在main函数中,我们首先构建了一个有向图,然后使用graphToRegex函数将其转换为正规表达式,并使用regexToDFA函数将其转换为等价的DFA。最后,我们比较了两个DFA是否等价,如果等价,则表示正规表达式正确。
阅读全文
相关推荐
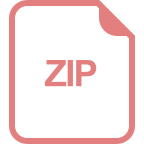
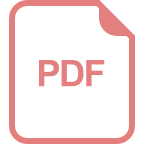
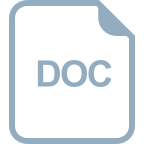
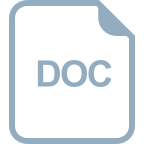
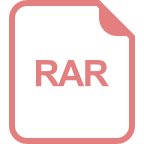
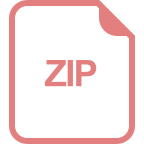
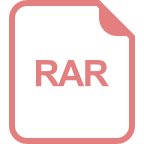
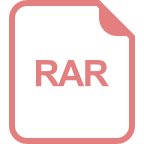
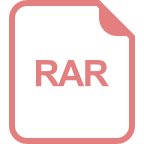
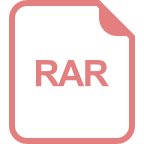
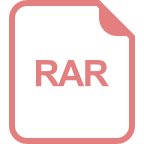
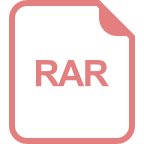
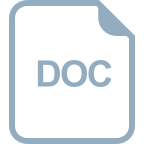
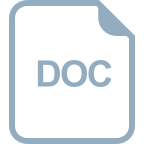
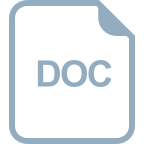
