给你两个长度为 n 、下标从 0 开始的整数数组 nums1 和 nums2 ,另给你一个下标从 1 开始的二维数组 queries ,其中 queries[i] = [xi, yi] 。 对于第 i 个查询,在所有满足 nums1[j] >= xi 且 nums2[j] >= yi 的下标 j (0 <= j < n) 中,找出 nums1[j] + nums2[j] 的 最大值 ,如果不存在满足条件的 j 则返回 -1 。 返回数组 answer ,其中 answer[i] 是第 i 个查询的答案。
时间: 2024-03-24 14:40:14 浏览: 99
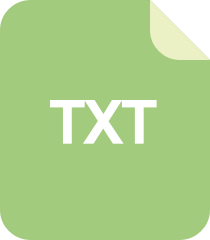
给定一个整数数组 nums 和一个目标值 target,请在数组中找出和为目标值的那两个整数,并返回它们的数组下标
这个问题可以使用二分查找和前缀最大值来解决。具体步骤如下:
1. 对 nums1 和 nums2 分别按照从大到小的顺序排序。
2. 对 nums2 计算前缀最大值数组 prefix_max2,其中 prefix_max2[i] 表示 nums2 中前 i 个数的最大值。
3. 对于每个查询 [xi, yi],在 nums1 中二分查找第一个大于等于 xi 的数的下标 index,如果找不到则返回 -1。如果找到了,则在 nums2 中查找第一个大于等于 yi 的数的下标 j,如果找不到则返回 -1。如果找到了,则最大的 nums1[j] + nums2[j] 就是 nums1[index] + prefix_max2[j]。
4. 将每个查询的结果保存到 answer 数组中并返回。
下面是实现这个算法的 Python 代码:
```python
from typing import List
def max_sum(nums1: List[int], nums2: List[int], queries: List[List[int]]) -> List[int]:
n = len(nums1)
nums1.sort(reverse=True)
nums2.sort(reverse=True)
prefix_max2 = [0] * (n + 1)
for i in range(n):
prefix_max2[i+1] = max(prefix_max2[i], nums2[i])
answer = []
for xi, yi in queries:
index = bisect_left(nums1, xi)
if index == n or nums2[0] < yi:
answer.append(-1)
else:
j = bisect_left(nums2, yi)
if j == n or nums1[index] + prefix_max2[j] < nums1[0] + nums2[0]:
answer.append(-1)
else:
answer.append(nums1[index] + prefix_max2[j])
return answer
```
其中 bisect_left 函数是 Python 内置的二分查找函数。
阅读全文
相关推荐
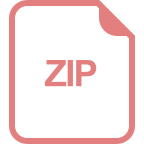
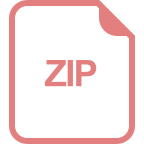












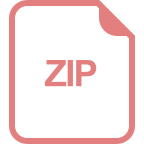
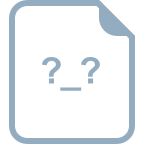