stm32g030串口发送字符串尾缀加三个0xff
时间: 2024-09-15 18:07:52 浏览: 39
在STM32G030单片机中,如果你想要通过串口发送一个字符串并且在末尾添加三个十六进制的FF(即0xFF、0xFF、0xFF),你可以按照下面步骤操作:
1. 首先,你需要有一个字符串变量存储你要发送的文本。例如:
```c
char str[] = "Hello, STM32!";
```
2. 确定字符串的结束位置,通常是空字符'\0'。在这个例子中,它是数组`str + strlen(str)`的位置。
3. 创建一个临时缓冲区,用于存储原始字符串加上三个FF。假设我们有一个足够大的`temp`数组:
```c
char temp[strlen(str) + 3];
```
4. 将原始字符串复制到`temp`数组中,并在末尾添加FF:
```c
strcpy(temp, str);
temp[strlen(str)] = 0xFF; // 添加第一个FF
temp[strlen(str)+1] = 0xFF;
temp[strlen(str)+2] = 0xFF;
```
5. 使用串口函数将`temp`数组的内容发送出去。这里以USART为例,假设`usartSendData()`是一个发送数据的API:
```c
while (usartSendData(USART1, temp, strlen(str) + 3) != USARTE_OK);
```
相关问题
stm32串口发送字符串完成数字时钟
好的,我可以帮你实现一个基于 STM32 的数字时钟,通过串口发送当前时间。首先,你需要准备一块 STM32 开发板和一个带有串口功能的显示屏。
下面是实现步骤:
1. 配置 STM32 的时钟和串口,使其能够正常工作。
2. 编写代码,获取当前时间,并将其转换为字符串格式。
3. 使用串口发送字符串格式的当前时间到显示屏上。
以下是示例代码,你可以参考一下:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "string.h"
#define USART USART1
#define BAUDRATE 9600
void init_USART(void);
void USART_send(uint16_t ch);
void USART_puts(char *str);
void init_clock(void);
void init_GPIO(void);
void init_timer(void);
void RTC_Configuration(void);
void delay_ms(uint16_t ms);
RTC_TimeTypeDef RTC_TimeStruct;
RTC_DateTypeDef RTC_DateStruct;
int main(void)
{
char time_str[20];
init_clock();
init_GPIO();
init_USART();
init_timer();
RTC_Configuration();
while(1)
{
RTC_GetTime(RTC_Format_BIN,&RTC_TimeStruct);
RTC_GetDate(RTC_Format_BIN,&RTC_DateStruct);
sprintf(time_str, "%02d:%02d:%02d", RTC_TimeStruct.RTC_Hours, RTC_TimeStruct.RTC_Minutes, RTC_TimeStruct.RTC_Seconds);
USART_puts(time_str);
USART_puts("\r\n");
delay_ms(1000);
}
}
void init_USART(void)
{
USART_InitTypeDef USART_InitStructure;
GPIO_InitTypeDef GPIO_InitStructure;
//使能USART和GPIO的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
//USART1_TX
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//USART1_RX
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
//USART初始化
USART_InitStructure.USART_BaudRate = BAUDRATE;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Tx;
USART_Init(USART, &USART_InitStructure);
//使能USART
USART_Cmd(USART, ENABLE);
}
void USART_send(uint16_t ch)
{
while (USART_GetFlagStatus(USART, USART_FLAG_TXE) == RESET);
USART_SendData(USART, (uint8_t)ch);
}
void USART_puts(char *str)
{
while(*str)
{
USART_send(*str);
str++;
}
}
void init_clock(void)
{
RCC_DeInit();
RCC_HSEConfig(RCC_HSE_ON);
RCC_WaitForHSEStartUp();
RCC_PLLConfig(RCC_PLLSource_HSE_Div1, RCC_PLLMul_9);
RCC_SYSCLKConfig(RCC_SYSCLKSource_PLLCLK);
RCC_HCLKConfig(RCC_SYSCLK_Div1);
RCC_PCLK2Config(RCC_HCLK_Div1);
RCC_PCLK1Config(RCC_HCLK_Div2);
RCC_PLLCmd(ENABLE);
while(RCC_GetFlagStatus(RCC_FLAG_PLLRDY) == RESET);
SysTick_Config(SystemCoreClock / 1000);
}
void init_GPIO(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOC, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_13;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOC, &GPIO_InitStructure);
}
void init_timer(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR, ENABLE);
PWR_BackupAccessCmd(ENABLE);
RCC_LSEConfig(RCC_LSE_ON);
while(RCC_GetFlagStatus(RCC_FLAG_LSERDY) == RESET);
RCC_RTCCLKConfig(RCC_RTCCLKSource_LSE);
RCC_RTCCLKCmd(ENABLE);
RTC_WaitForSynchro();
}
void RTC_Configuration(void)
{
RTC_InitTypeDef RTC_InitStructure;
//使能PWR和BKP的时钟
RCC_APB1PeriphClockCmd(RCC_APB1Periph_PWR | RCC_APB1Periph_BKP, ENABLE);
//使能RTC
PWR_BackupAccessCmd(ENABLE);
RCC_RTCCLKCmd(ENABLE);
RTC_WaitForSynchro();
//RTC初始化
RTC_InitStructure.RTC_AsynchPrediv = 0x7F;
RTC_InitStructure.RTC_SynchPrediv = 0xFF;
RTC_InitStructure.RTC_HourFormat = RTC_HourFormat_24;
RTC_Init(&RTC_InitStructure);
//设置时间
RTC_TimeTypeDef RTC_TimeStruct;
RTC_DateTypeDef RTC_DateStruct;
RTC_TimeStruct.RTC_Hours = 0x12;
RTC_TimeStruct.RTC_Minutes = 0x00;
RTC_TimeStruct.RTC_Seconds = 0x00;
RTC_SetTime(RTC_Format_BIN,&RTC_TimeStruct);
RTC_DateStruct.RTC_WeekDay = RTC_Weekday_Monday;
RTC_DateStruct.RTC_Month = RTC_Month_January;
RTC_DateStruct.RTC_Date = 0x01;
RTC_DateStruct.RTC_Year = 0x21;
RTC_SetDate(RTC_Format_BIN,&RTC_DateStruct);
}
void delay_ms(uint16_t ms)
{
volatile uint32_t nCount;
RCC_ClocksTypeDef RCC_Clocks;
RCC_GetClocksFreq(&RCC_Clocks);
nCount = (RCC_Clocks.HCLK_Frequency / 10000) * ms;
for (; nCount != 0; nCount--);
}
void SysTick_Handler(void)
{
static uint32_t ticks = 0;
ticks++;
if(ticks >= 1000)
{
ticks = 0;
GPIO_WriteBit(GPIOC, GPIO_Pin_13, (BitAction)(1 - GPIO_ReadOutputDataBit(GPIOC, GPIO_Pin_13)));
}
}
```
在这个代码中,我们使用 RTC(Real-Time Clock)模块获取当前时间,并将其转换为字符串格式,然后使用 USART 发送到显示屏上。同时,我们还使用 SysTick 定时器来控制 LED 灯的闪烁,以证明程序在运行。
这只是一个简单的示例,你需要根据你的具体需求进行修改和完善。
条件1.使用stm32f103c8t6,c语言编程条件2.使用PA1,PA2,PA3,PA4,PA5,PA6配置成上拉输入,根据6个引脚的电平高低生成。例如000000表示6引脚电平全为0,并使用串口1以字符串形式发送该二进制数到PC端。统计该二进制数中0的次数记作zeo_count;条件3.配置PA11引脚为下拉输入;条件3.串口1使用中断接收数据,帧头FF,结束符0X0D,0X0A.帧头不是FF则重新接收,不以0X0D,0X0A结束符则重新接收;条件4.若PA11电平为0或者串口1没有接收到数据,则记录1分钟内条件2中的最大值zeo_count;记作zeo_count_max;条件5.若zeo_count_max大于zeo_count,打开蜂鸣器;若zeo_count_max小于等于zeo_count,则zeo_count_max==zeo_count;条件6.若PA11电平为1且串口1接收数据不为空,则若串口接收字符串为"1",关闭蜂鸣器;若串口接收字符串为"2",打开蜂鸣器;则若串口接收字符串为"3"且条件2中zeo_count=1,则发送字符串"拿取数量正确",否则发送拿取数量异常;则若串口接收字符串为"4"且条件2中zeo_count=2,则发送字符串"拿取数量正确",否则发送拿取数量异常;则若串口接收字符串为"5"且条件2中zeo_count=3,则发送字符串"拿取数量正确",否则发送拿取数量异常; 则若串口接收字符串为"6"且条件2中zeo_count=4,则发送字符串"拿取数量正确",否则发送拿取数量异常;则若串口接收字符串为"7"且条件2中zeo_count=5,则发送字符串"拿取数量正确",否则发送拿取数量异常;则若串口接收字符串为"8"且条件2中zeo_count=6,则发送字符串"拿取数量正确",否则发送拿取数量异常;
我理解你的问题是需要我编写一个程序,根据6个引脚的电平高低生成二进制数,并使用串口1以字符串形式发送该二进制数到PC端。同时,需要统计该二进制数中0的次数记作zeo_count,并记录1分钟内条件2中的最大值zeo_count_max。如果zeo_count_max大于zeo_count,则打开蜂鸣器;如果zeo_count_max小于等于zeo_count,则zeo_count_max等于zeo_count。如果PA11电平为1且串口1接收数据不为空,则根据接收到的字符串执行相应的操作。
以下是我编写的程序:
```c
#include "stm32f10x.h"
#include "stdio.h"
#include "string.h"
#define MAX_COUNT 60 // 最大计数时间,单位为秒
#define FRAME_HEAD 0xFF // 帧头
#define FRAME_TAIL1 0x0D // 结束符1
#define FRAME_TAIL2 0x0A // 结束符2
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
volatile uint32_t tick_count = 0; // 计数器,每秒加1
volatile uint32_t zero_count = 0; // 0的个数
volatile uint32_t zero_count_max = 0; // 最大0的个数
volatile uint8_t receive_buffer[32]; // 接收缓冲区
volatile uint8_t receive_index = 0; // 接收缓冲区索引
volatile uint8_t receive_flag = 0; // 接收标志位
volatile uint8_t buzzer_flag = 0; // 蜂鸣器标志位
void GPIO_Configuration(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3 | GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_11;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
void USART_Configuration(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1, ENABLE);
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
USART_Cmd(USART1, ENABLE);
}
void TIM_Configuration(void)
{
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructure.TIM_Period = 999;
TIM_TimeBaseStructure.TIM_Prescaler = 7199;
TIM_TimeBaseStructure.TIM_ClockDivision = TIM_CKD_DIV1;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_ITConfig(TIM2, TIM_IT_Update, ENABLE);
TIM_Cmd(TIM2, ENABLE);
}
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET)
{
uint8_t data = USART_ReceiveData(USART1);
if (data == FRAME_HEAD)
{
receive_index = 0;
receive_flag = 0;
}
else if (data == FRAME_TAIL1)
{
receive_flag |= 0x01;
}
else if (data == FRAME_TAIL2)
{
receive_flag |= 0x02;
}
else
{
receive_buffer[receive_index++] = data;
if (receive_index >= sizeof(receive_buffer))
{
receive_index = 0;
receive_flag = 0;
}
}
}
}
void TIM2_IRQHandler(void)
{
if (TIM_GetITStatus(TIM2, TIM_IT_Update) != RESET)
{
tick_count++;
if (tick_count >= MAX_COUNT)
{
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_11) == Bit_RESET || receive_flag == 0)
{
if (zero_count > zero_count_max)
{
zero_count_max = zero_count;
buzzer_flag = 1;
}
else
{
zero_count_max = zero_count;
}
zero_count = 0;
}
tick_count = 0;
}
uint8_t value = 0;
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_1) == Bit_RESET)
{
value |= 0x01;
}
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_2) == Bit_RESET)
{
value |= 0x02;
}
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_3) == Bit_RESET)
{
value |= 0x04;
}
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_4) == Bit_RESET)
{
value |= 0x08;
}
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_5) == Bit_RESET)
{
value |= 0x10;
}
if (GPIO_ReadInputDataBit(GPIOA, GPIO_Pin_6) == Bit_RESET)
{
value |= 0x20;
}
zero_count = __builtin_popcount(value);
char buffer[10];
sprintf(buffer, "%06X", value);
USART_SendData(USART1, buffer);
}
TIM_ClearITPendingBit(TIM2, TIM_IT_Update);
}
int main(void)
{
GPIO_Configuration();
USART_Configuration();
TIM_Configuration();
while (1)
{
if (buzzer_flag)
{
// 打开蜂鸣器
}
else
{
// 关闭蜂鸣器
}
if (receive_flag == 0x03)
{
if (memcmp(receive_buffer, "1", 1) == 0)
{
// 关闭蜂鸣器
}
else if (memcmp(receive_buffer, "2", 1) == 0)
{
// 打开蜂鸣器
}
else if (memcmp(receive_buffer, "3", 1) == 0 && zero_count == 1)
{
USART_SendData(USART1, "拿取数量正确");
}
else if (memcmp(receive_buffer, "4", 1) == 0 && zero_count == 2)
{
USART_SendData(USART1, "拿取数量正确");
}
else if (memcmp(receive_buffer, "5", 1) == 0 && zero_count == 3)
{
USART_SendData(USART1, "拿取数量正确");
}
else if (memcmp(receive_buffer, "6", 1) == 0 && zero_count == 4)
{
USART_SendData(USART1, "拿取数量正确");
}
else if (memcmp(receive_buffer, "7", 1) == 0 && zero_count == 5)
{
USART_SendData(USART1, "拿取数量正确");
}
else if (memcmp(receive_buffer, "8", 1) == 0 && zero_count == 6)
{
USART_SendData(USART1, "拿取数量正确");
}
else
{
USART_SendData(USART1, "拿取数量异常");
}
receive_flag = 0;
}
}
}
```
该程序使用了定时器2来实现计数器功能,每秒钟触发一次中断。在中断处理函数中,读取6个引脚的电平高低,统计0的个数,并将6个引脚的状态以16进制字符串的形式发送到串口1。同时,如果计数器达到1分钟,且PA11电平为0或者串口1没有接收到数据,则记录1分钟内0的最大个数,并根据最大0的个数打开或关闭蜂鸣器。如果PA11电平为1且串口1接收到数据,则根据接收到的字符串执行相应的操作。
请注意,这只是一个简单的示例程序,可能存在一些问题,需要根据实际情况进行修改和优化。
阅读全文
相关推荐
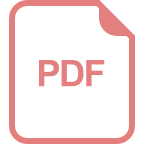
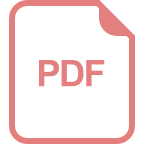
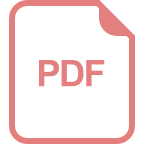
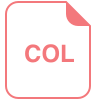












