A*算法计算11*13迷宫路径的全部代码
时间: 2024-10-28 10:12:09 浏览: 10
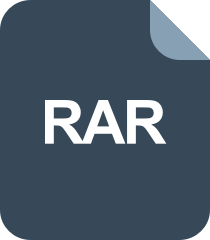
A*算法学习(python代码实现)
A*算法需要一个网格化的迷宫表示,这里假设迷宫是一个二维列表,1表示墙,0表示可以通过的空格。下面是一个简单的Python代码示例,展示了如何使用A*算法在给定的11x13迷宫中寻找从起点到终点的路径:
```python
import heapq
# 定义迷宫数据
maze = [
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1], # 起点 (0, 0)
[1, 0, 1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1], # 终点 (2, 9)
[1, 0, 0, 0, 1, 1, 1, 0, 0, 0, 1],
[1, 0, 0, 0, 1, 1, 0, 0, 0, 0, 1],
[1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 1],
[1, 0, 0, 1, 1, 1, 0, 1, 1, 0, 1],
[1, 0, 0, 0, 0, 1, 1, 0, 0, 1, 1],
[1, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
]
# 计算启发式函数值,例如曼哈顿距离
heuristic = lambda pos, goal: abs(pos[0] - goal[0]) + abs(pos[1] - goal[1])
# 函数定义
def a_star_search(maze, start, end):
frontier = [(0, start)]
came_from = {}
cost_so_far = {start: 0}
while frontier:
current_cost, current_pos = heapq.heappop(frontier)
if current_pos == end:
break
for next_pos in get_neighbors(maze, current_pos):
new_cost = current_cost + 1
if next_pos not in cost_so_far or new_cost < cost_so_far[next_pos]:
cost_so_far[next_pos] = new_cost
priority = new_cost + heuristic(next_pos, end)
heapq.heappush(frontier, (priority, next_pos))
came_from[next_pos] = current_pos
path = []
current_pos = end
while current_pos != start:
path.append(current_pos)
current_pos = came_from.get(current_pos)
path.append(start) # 添加起始点
path.reverse() # 反转路径顺序
return path[::-1] # 返回路径
# 获取邻居函数
def get_neighbors(maze, pos):
row, col = pos
neighbors = [(row - 1, col), (row + 1, col), (row, col - 1), (row, col + 1)]
valid_neighbors = [(r, c) for r, c in neighbors if 0 <= r < len(maze) and 0 <= c < len(maze[0]) and maze[r][c] == 0]
return valid_neighbors
# 主程序
path = a_star_search(maze, (0, 0), (2, 9))
print("Path from start to end:", path)
```
这个代码将返回从起点到终点的最短路径。如果迷宫中不存在这样的路径,`a_star_search`函数会返回None。运行此代码前,请确保输入正确的迷宫矩阵。
阅读全文
相关推荐
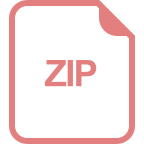
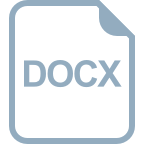
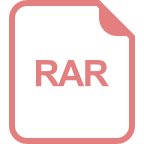
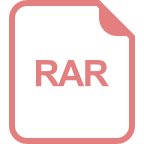
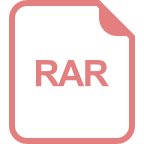
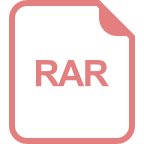
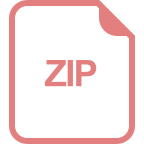
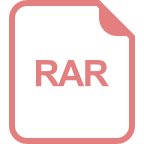
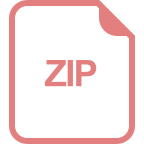
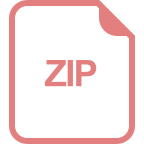
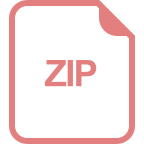
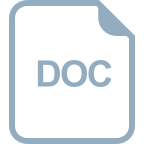
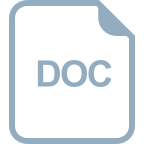
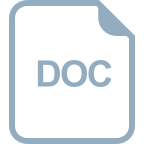



